SQL Learning 2 - Query Introduction
Made by Mike_Zhang
1. Abstract
Continue with the previous post where we have created the table and discussed how to manipulate them. Now we can query data inside the table with SQL’s Query Mechanics with SELECT
Statements, which can help us to retrieve, join, filter, group and sort data from the table1.
SELECT
Statements have several Clause (parts). There are six commonly used clauses in MySQL:
Clause | Description |
---|---|
SELECT |
Determines which of all possible columns should be indicated in the query’s result set. |
FROM |
Defines the tables used by the query, with the ability of joining the tables together. |
WHERE |
Filters out unwanted rows from the result set. |
GROUP BY |
Groups rows together based on the common value of columns. |
HAVING |
Filters out unwanted groups from the result set. |
ORDER BY |
Sorts results set using either raw column data or expressions of them. |
2. SELECT
Clause
SELECT
Clause determines which of all possible columns should be indicated in the query’s result set.
2.1 Basic Syntax
- Select all columns from a table (indicated by
*
):1
2SELECT *
FROM [table_name]; - Select specific columns from a table (explicitly indicate names):
1 |
|
[Example]
1 |
|
2.2 Additional Columns
The SELECT clause’s result set is not limited by the only columns in the table indicated by FROM
. Users are able to add additional columns into the result set without affecting data in the real table.
Four types of additional columns added by users:
- Literals: numbers, strings,…;
- Expressions: such as
language.language_id + 1
; - Build-in function calls: such as
upper()
; - User-defined function calls;
[Example]
1 |
|
2.3 Column Aliases
- SQL adds label to each column from the table in the result set, such as
language_id
. - However, users can add their own label to additional columns, which are Column Aliases.
Syntax:
1 |
|
or
1 |
|
- where
AS
keyword is optional, which is used for easy reading and understanding
[Example]
1 |
|
2.4 Distinct Data
- To get the distinct set of result, no more duplicated data, like
ID
.
Syntax:
Just add DISTINCT
keyword after the SELECT
keyword.
1 |
|
[Example]
- WITHOUT
DISTINCT
keyword:
1 |
|
- WITH
DISTINCT
keyword:
1 |
|
Remainder:
- The opposite of
DISTINCT
isALL
keyword, which is the default and NO need to be explicitly added into statements. - Using
DISTINCT
to get the result requires sorting the result set, which is time consuming. Users should rethink before using it.
3. FROM
Clause
FROM
Clause defines the tables used by the query, with the ability of joining the tables together.
3.1 Basic Syntax
1 |
|
[Example]
1 |
|
3.2 Table Types
Four types of tables can be used in the FROM
clause:
- Permanent table:
- Commonly used table created using
CREATE TABLE
statement.
- Commonly used table created using
Derived table:
Generated by subquery (a query inside another query) within the
FROM
clause, to generate a derived table and visible to all other query clauses inFROM
clause:1
2
3
4
5SELECT d.name
FROM
(SELECT name
FROM customer
) AS d;- Where
(SELECT name FROM customer)
is a derived table with aliasd
. - Columns in the derived table can be referenced by table alias, such as
d.name
.
- Temporary table:
- It is a volatile, temporary table, like a local variable, which looks like a permanent table. But will disappear when database session closed.
- Syntax:
1
2
3
4CREATE TEMPORARY TABLE [table_name](
[column_name] [data_type] [column_constraint],
...
) - Then can be manipulated and queried like a permanent table.
- Views / Virtual table:
- No data associated with a virtual table, only references.
- Syntax:
1
2
3CREATE VIEW [view_name] AS
SELECT [column_name], ..., [column_name]
FROM [table_name];- After a view is created, NO additional data generated and stored in the database, only references.
- View can be used by the
FROM
clause:1
2SELECT *
FROM [view_name];- WThe final real query to the views will NOT be generated until it is executed by a query.
- Usage:
- The view can hide columns from users, as well as simplify complex database design.
3.3 Table Links
More than one tables can appear in the FROM
clause, then these tables can be linked/joined together based on the relational design.
Syntax:
1 |
|
[Example]
1 |
|
Where
- Table
customer
andrental
are joined together within thrFROM
clause. - The join condition is ON
customer.customer_id = rental.customer_id
for the primary key.
3.4 Table Aliases
Two ways to reference a table and its columns outside the FROM
clause:
- Using the entire table name
- such as
customer.customer_id
, or
- such as
- Assign tables aliases, then use the alias as reference name:
FROM customer AS c
orFROM customer c
(Assign table alias asc
)c.customer_id
(Reference the columncustomer_id
of the tablec
)
4. WHERE
Clause
WHERE
clause filters out unwanted rows from the result set.
Syntax:
1 |
|
Where
conditions
is a logical expression that combinesand
,or
, andnot
operators as well as their statements.- Separate groups of conditions using parentheses.
[Example]
1 |
|
5. GROUP BY
and HAVING
Clause
GROUP BY
Clause groups rows together based on the common value of columns.HAVING
Clause filters out unwanted groups from the result set.
Syntax:
1 |
|
[Example]
1 |
|
6. ORDER BY
Clause
ORDER BY
Clause sorts results set using either raw column data or expressions of them.
6.1 Syntax
1 |
|
Where
[column_name_1], .., [column_name_n]
is the sorting criteria, can be extended to more than one condition;ASC/DESC
is the sort order:ASC
is ascending order, default;DESC
is descending order.
[Example]
1 |
|
6.2 Sort via Column Position
Rather than using column names, the target column can be indicated by its position in SELECT
clause.
Syntax:
1 |
|
[Example]
1 |
|
Where
2
refers to the second column in theSELECT language_id, name
, which isname
.
But it is NOT recommended to use this method because columns in SELECT
statement may be changed frequently, so do their positions.
References
1. A. Beaulieu, LEARNING SQL: master sql fundamentals. S.L.: O’reilly Media, Inc, Usa, 2020. ↩
Outro
This post has just introduced the Query Primer in SQL. More details will be discussed in the following posts. See you next time!
初次接触SQL,相关的知识会继续学习,继续更新.
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

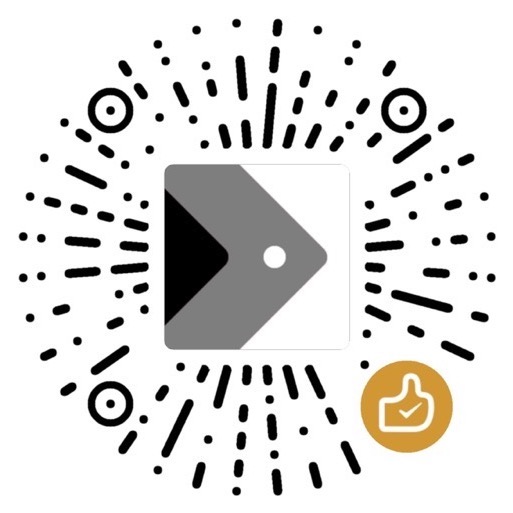