SQL Learning 1 - Intro, Data Types & Table
Made by Mike_Zhang
1. Abstract
This post assumes that you have already known the basics of relational database model. This post will introduce the basic concepts of SQL1, some SQL syntax, SQL data types in MySQL, as well as Table in MySQL. Regarding the SQL basic grammar (e.g. Query statements), the next post will have more detailed discussions.
2. SQL Introduction
SQL is a programming language for manipulating data in a relational database as well as various database technologies 1.
According to Beaulieu1, “SQL” is NOT an acronym for “Structured Query Language”, and we should say its three letters (i.e. “S”, “Q”, “L”) or the word sequel.
However, I think considering it as an acronym is also appropriate as long as it is reasonable.
Three parts of SQL language:
- SQL schema statements
- Define the data types and structures in the database;
- SQL data statements
- Manipulate the data in the defined database;
- SQL transaction statements
- Begin, end, and roll back transactions.
(This SQL Learning Topic will mainly focus on the first two parts of SQL language.)
[Example]
Using SQL schema statement to create a table named “person”, having person_id
, fname
, and birth_date
as columns where person_id
is an primary key of the table.
1 |
|
[Example]
Using SQL data statements to modify and insert a person’s data into the person
table.
1 |
|
As well as to retrieve data from the person
table.
1 |
|
Output:
1 |
|
SQL is a nonprocedural language 1.
Procedural language, like Java, Python, etc, defines the desired results and the algorithms or processes.
While SQL as a nonprocedural language, only defines the desired results (output), but leaves the algorithms or processes to others, which means that we do not need to concern how to
CREATE
orSELECT
the data.
Data structure or data type is one of the most important concepts in almost all programming language. So do SQL. Before SQL making changes on the database (tables), first we need tables. So we have to define what kind of data we want to store in the tables.
Next we are going to discuss the data types in SQL, defined by the SQL schema statements.
3. MySQL Data Types
Before apply SQL to the database, first we need database and its serves, like MySQL, Oracle Database, SQL Server, DB2, etc. MySQL will be used as the example to implement the SQL statements.
Three major data types in MySQL:
- Character Data
- Numeric Data
- Temporal Data
3.1 Character Data
Character data is stored in fixed-length strings or variable-length strings.
- fixed-length strings have right-padded spaces with same numbers of characters;
- variable-length strings have NO right-padded spaces with different numbers of characters.
[Example]
Storing string up to 10 characters in a fixed-length string column.
1 |
|
- Maximum length of
CHAR
is 255 bytes; - Maximum length of
VARCHAR
is 65,535 bytes.
All string data type in MySQL 2:
CHAR
,VARCHAR
,BINARY
,VARBINARY
,BLOB
,TEXT
,ENUM
, andSET
.
Character sets in MySQL:
1 |
|
3.2 Numeric Data
3.2.1 Integer
MySQL integer types 1:
Type | Singed Range | Unsigned Range | Bytes |
---|---|---|---|
TINYINT |
$-128$ to $127$ | $0$ to $255$ | 1 |
SMALLINT |
$-32768$ to $32767$ | $0$ to $65535$ | 2 |
MEDIUMINT |
$-8388608$ to $8388607$ | $0$ to $16777215$ | 3 |
INT |
$-2147483648$ to $2147483647$ | $0$ to $4294967295$ | 4 |
BIGINT |
$-2^{63}$ to $2^{63}-1$ | $0$ to $2^{64}-1$ | 8 |
3.2.2 Floating-point
MySQL floating-point types 1:
Type | Range |
---|---|
FLOAT(p, s) |
$−3.402823466E+38$ to $−1.175494351E-38$ and $1.175494351E-38$ to $3.402823466E+38$ |
DOUBLE(p, s) |
$−1.7976931348623157E+308$ to $−2.2250738585072014E-308$ and $2.2250738585072014E-308$ to $1.7976931348623157E+308$ |
Where,
p
for precision, indicating the total number of digits to the left and right of the decimal point;s
for scale, indicating the number of digits to the right of the decimal point.
3.3 Temporal Data
This is the data type for storing date and time values.
MySQL temporal types 1:
Type | Format | Range |
---|---|---|
DATE |
YYYY-MM-DD | $1000-01-01$ to $9999-12-31$ |
DATETIME |
YYYY-MM-DD HH:MI:SS | $1000-01-01 00:00:00.000000$ to $9999-12-31 23:59:59.999999$ |
TIMESTAMP |
YYYY-MM-DD HH:MI:SS | $1000-01-01 00:00:00.000000$ to $9999-12-31 23:59:59.999999$ |
YEAR |
YYYY | $1901$ to $2155$ |
TIME |
HHH:MI:SS | $-838:59:59.000000$ to $838:59:59.000000$ |
Date format components 1:
Component | Definition | Range |
---|---|---|
YYYY |
Year | $1000$ to $9999$ |
MM |
Month | $01$ to $12$ |
DD |
Day | $01$ to $31$ |
HH |
Hour | $00$ to $23$ |
HHH |
Hours (elapsed) | $-838$ to $838$ |
MI |
Minute | $00$ to $59$ |
SS |
Second | $00$ to $59$ |
4. MySQL Table
After the definition of the above data types, we can define the table by using these data types.
[Example]
We are going to design a table storing personal information, including
info | Type | Values |
---|---|---|
person_id | SMALLINT |
|
first_name | VARCHAR(20) |
|
last_name | VARCHAR(20) |
|
eye_color | CHAR(2) |
BR , BL , GR |
birth_date | DATE |
|
street | VARCHAR(40) |
|
city | VARCHAR(40) |
|
state | VARCHAR(40) |
|
country | VARCHAR(40) |
|
postal_code | VARCHAR(20) |
This design is based on the concept of normalization, which will be discussed in the further posts.
4.1 Creating Tables
- Using SQL Schema Statements to create tables.
1 |
|
- Turn on the
auto-increment
feature primary key column.
1 |
|
describe
ordesc
command can be used to check the table definition.
1 |
|
Finally, the person
table is created. Now we can manipulate the table.
4.2 Modifying Tables
4.2.1 Inserting
Three components in the insert statement:
- The name of the table to insert into;
- The name of the columns to insert into;
- The values to insert into the columns.
There is no need to provide data to every column for each inserted data unless the column defined as not null.
For theauto_increment
primary key, just provide anull
value for increasing automatically.
- Using SQL data statements to insert data into the table.
1 |
|
[Example]
1 |
|
- Using
SELECT
to check the table:
1 |
|
4.2.2 Updating
- Using SQL data statements to update data into the table.
1 |
|
[Example]
1 |
|
- Using
SELECT
to check the table:
1 |
|
4.2.3 Deleting
- Using SQL data statements to update data into the table.
1 |
|
[Example]
1 |
|
- Using
SELECT
to check the table:
1 |
|
References
1. A. Beaulieu, LEARNING SQL: master sql fundamentals. S.L.: O’reilly Media, Inc, Usa, 2020. ↩
2. “MySQL :: MySQL 8.0 Reference Manual :: 11.3.1 String Data Type Syntax,” dev.mysql.com. https://dev.mysql.com/doc/refman/8.0/en/string-type-syntax.html (accessed Aug. 09, 2022). ↩
Outro
In the next post, we will discuss SQL’s Query statement, which is the SELECT
statement, as well as filtering.
初次接触SQL,相关的知识会继续学习,继续更新.
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

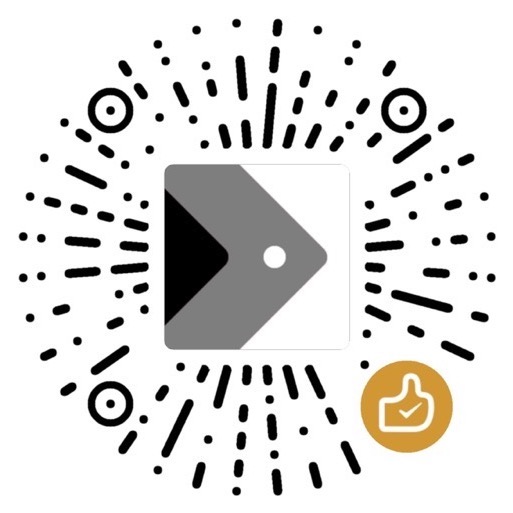