Sorting Algorithm Introduction - 排序算法简介
Made by Mike_Zhang
数据结构与算法主题:
1 Introduction to Sorting Algorithm
Sorting is a process of arranging a sequence of elements into some logical order (e.g. increasing or decreasing order).
Nowadays, most of programming languages and computer systems have implementations of sorting algorithms.
But, it is still very important to know and study how to implement sorting algorithms as
- Sorting algorithm can be used to solve other problems in a similar way;
- Sorting algorithm can be the first step of solving other problems;
- Sorting algorithm analysis is a good practice of algorithm performance analysis.
Let’s introduce some popular sorting algorithms: Bubble Sort, Selection Sort, Insertion Sort, Merge Sort, Quick Sort, Counting Sort, Heap Sort, etc.
(Assume all resulting arrays are sorted in ascending order.)
2 Bubble Sort
冒泡排序
Algorithm:
- Starting from the first element, compare the first adjacent elements of the array, if the first element is larger than the second element, swap the two elements;
- Repeat doing step 1 for each pair of adjacent elements from the first element to the last element, end up with the biggest element in the last position.
- Repeat doing above steps for all element except the last element;
- Each time, the relatively biggest element will be moved towards the end of the array, the array is sorted when no swap is made.
Illustration:
Source: VisuAlgo
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n^2)$
Worst time complexity: $O(n^2)$
3 Selection Sort
选择排序
- Starting from the first element, find the smallest element in the array, swap it with the first element;
- Then find the next smallest element in the array, swap it with the second element;
- Repeat in the above way until the array is sorted.
Each time we select the smallest element in remaining unsorted array, and move it to the beginning of the unsorted part of array (or end of sorted part).
Illustration:
Source: VisuAlgo
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n^2)$
Worst time complexity: $O(n^2)$
4 Insertion Sort
插入排序
Algorithm:
- Assume the first element is already sorted, start from the second element;
- Scan the sorted elements($S$) before the current element($C$) (left in illustration) from the end to the beginning;
- If the element $C$ is smaller than the element $S$, move the scanner to next position;
- Repeat step 3 until the element $C$ is larger than or equal to the element $S$, then insert the element $C$ into the position.
- Repeat step 2-4 until all elements are sorted.
Illustration:
Source: VisuAlgo
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n^2)$
Worst time complexity: $O(n^2)$
5 Merge Sort
并归排序
Algorithm:
A classic application of Divide and Conquer(分治法) algorithm.
- Divide the array into two halves;
- Recursively sort the two halves;
- Merge the two sorted halves into one sorted array.
Illustration:
Source: VisuAlgo
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n log(n))$
Worst time complexity: $O(n log(n))$
6 Quick Sort
快速排序
Algorithm:
A classic application of Divide and Conquer(分治法) algorithm.
- Choose a pivot element (start from the first element);
- Compare the pivot element with the rest of the elements;
- Find all elements that are smaller than the pivot element, rearrange them in the left part of the array;
- Find all elements that are larger than the pivot element, rearrange them in the right part of the array;
- Now, the pivot element is in the correct position;
- Repeat step 2-5 for the left and right parts of the array recursively.
Illustration:
Source: Quicksort - Algorithm Visualizer
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n log(n))$
Worst time complexity: $O(n^2)$
7 Counting Sort
计数排序
Very interesting sorting algorithm. More details will be discussed in later articles.
Algorithm:
- Find the maximum element in the array (as value $M$);
- Build a count array with size $M + 1$ (as array $C$);
- Count the number of each element in unsorted array (as element $i$ appears $n$ times), store
C[i] = n
; - Accumulate each element in count array with the previous one (as element $i$ should appears in position of $(C[i - 1] + C[i])$) to get the final position of each element;
- Put all elements back to the array in the correct position (the position of element $i$ is $(C[i - 1] + C[i])$);
Illustration:
Source: VisuAlgo
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n+k)$
Worst time complexity: $O(n+k)$
8 Heap Sort
堆排序
Algorithm:
Very similar to selection sort, but instead of using linear scan to find the maximum element, we use heap data structure to find the maximum element.
Heap:
A binary tree whose root is the largest element in the tree, and the value of each vertex (except the root) is greater than the value of its child vertices.
Illustration:
Heap Sort - Algorithm Visualizations
Java implementation:
1 |
|
Test:
1 |
|
1 |
|
Average time complexity: $O(n log(n))$
Worst time complexity: $O(n log(n))$
9 Summary
Sort Algorithm | Average Time Complexity | Worst Time Complexity |
---|---|---|
Bubble Sort | $O(n^2)$ | $O(n^2)$ |
Selection Sort | $O(n^2)$ | $O(n^2)$ |
Insertion Sort | $O(n^2)$ | $O(n^2)$ |
Merge Sort | $O(n log(n))$ | $O(n log(n))$ |
Quick Sort | $O(n log(n))$ | $O(n^2)$ |
Counting Sort | $O(n+k)$ | $O(n+k)$ |
Heap Sort | $O(n log(n))$ | $O(n log(n))$ |
Source: Big-O Cheat Sheet
References
Algorithm Visualizer
Big-O Cheat Sheet
M. Goodrich, R. Tamassia, and A. O’reilly, Data Structures and Algorithms in Java, 6th Edition. John Wiley & Sons, 2014.
R. Sedgewick and K. Wayne, Algorithms. Upper Saddle River, Nj: Addison-Wesley, 2011.
十大经典排序算法大梳理 (动图+代码)
visualising data structures and algorithms through animation - VisuAlgo
写在最后
还有很多有趣的排序算法,如Bucket Sort, Radix Sort, Shell Sort,会继续学习,继续更新。
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

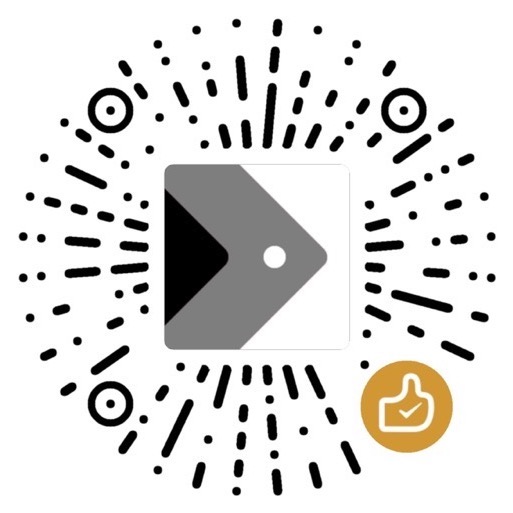