Linux Learning 3 - Shell Script - Variables, Assignments, Parameters and I/O
Made by Mike_Zhang
Unfold Linux Topics | 展开Linux主题 >
1 Introduction
- A script is a program that is interpreted instead of compiled. An interpreter takes each instruction in the script one at a time, translates it to an executable statement, and executes it;
- Shell Scripting is to produce code to automate Linux system tasks.
2 Create and Run
- Create a script file
1 |
|
- Write the script
- Every shell script must start with a comment that specifies the interpreter which should run the script.
- Bash:
#!/bin/bash
- Csh:
#!/bin/csh
- Tcsh:
#!/bin/tcsh
- Bash:
1 |
|
When in the vi window, press key
I
to edit, pressEsc
to exit edit mode, then type:wq
and pressEnter
to save and exit.
- Change the file permission
- The script file must also be executable. We will use permission 745, or alternatively 755.
1 |
|
- Run the script
- execute this script by entering:
1 |
|
- The start script outputs information to your terminal window.
echo
: output statement;#
: comment
1 |
|
Redirect the script’s output to a file:
1 |
|
output.txt
file:
1 |
|
3 Variables Assignments Parameters
3.1 Variables in Bash
Environment variables:
- because they have been exported, are accessible from within your script or from the command line and variables defined within your script that are only available in the script.
Variables:
- letters, numbers, and underscores(_);
- starts with a letter or underscore;
- case sensitive
- reserved words (e.g., if, then, while) are only recognizable if specified in lower case.
- variable names can be the same as reserved words such as if, but not recommended.
- Variables in Bash store ONLY strings. Numbers are treated as strings unless we specify that we want to interpret the value as a number;
3.2 Assignment Statements
1 |
|
- No spaces are allowed around the equal sign;
- Variable does NOT need to be declared;
- Value on the right hand side can be one of four things:
- A literal value such as Hello, “Hi there,” or 5
- The value stored in another variable, in which case the variable’s name on the right hand side of the assignment statement is preceded by a dollar sign as in
$FIRST_ NAME
or$X
- The result of an arithmetic or string operation
- The result returned from a Linux command
Quote mark:
- If the value is a string with no spaces, then quote marks may be omitted;
- If the value is a string with spaces, quote marks must be included.
[Example]
X=5
- X stores the string 5
NAME = Frank
- NAME stores Frank
NAME = "Frank Zappa"
- NAME stores Frank Zappa, the quote marks are required because of the space between Frank and Zappa
NAME = Frank Zappa
- An error arises because of the space, the Bash interpreter thinks that Zappa is a command, which does not exist
NAME = FrankZappa
- No quote marks are required here because there is no space, so in fact the literal value is really one string of 10 characters
NAME = ls *
- An error should arise as Bash tries to execute *, which is replaced by all of the entries in the current directory. If all of the entries in the directory are executable programs, this will result in all of these programs executing!
X = 1.2345
- X stores string 1.2345
To retrieve the value stored in a variable:
- Must precede the variable name with a
$
as in$NAME
or$X
. - However, the $ is NOT applied if the variable is enclosed in single quote marks (‘ ’).
- It is applied if the variable is enclosed in double quote marks (“ ”)
[Example]
NAME = "$FIRST_NAME $LAST_NAME"
- NAME stores Frank Zappa
NAME = '$FIRST_NAME $LAST_NAME'
- NAME stores
$FIRST_NAME
$LAST_NAME
NAME = $FIRST_NAME$LAST_NAME
- Name stores FrankZappa, notice that since there is no space on the right hand side of the assignment statement, we did not need to place
$FIRST_Name
$LAST_NAME
in quote marks
NAME = $FIRST_NAME $LAST_NAME
- Results in an error because there is a space and no quote marks
NAME = "FIRST_NAME LAST_NAME"
- NAME stores FIRST_NAME LAST_NAME because there is no
$
preceding the variable names
NAME = 'FIRST_NAME LAST_NAME'
- NAME stores FIRST_NAME LAST_NAME
To remove a value from a variable
- Assign it the
NULL
value:VARIABLE = NULL
- Having nothing on the right hand side of the equal sign:
VARIABLE =
- Use the
unset
command:unset VARIABLE
3.3 Executing Linux Commands
assignment statement: DATE = date
- sets the variable DATE to store the string date
- and sending the output to the terminal window
To execute and store the command ONLY, not output to the terminal window:
- place command within either ` ` marks or
$()
marks;
[Example]
1 |
|
- This instruction causes the Linux date command to execute
- NOT sending the output to the terminal window,
- the output is stored in the variable DATE.
[Example]
DATE = "Hello $FIRST_NAME, today’s date and time is $(date)"
- embed the entire right hand side in “” or ‘’ marks
If the item enclosed inside ` ` or $() is not a Linux command, an error will arise.
To declare a variable
1 |
|
- options and the initial value are optional
- Options are denoted
+
to turn an attribute of the variable off- and
−
to turn it on. - Option:
a
(array),f
(function),i
(integer),r
(read-only), andx
(exported).
[Example]
1 |
|
- create a read-only variable,
ARCH
, with the value 386 and export it beyond the current shell
3.4 Arithmetic Operations
Two ways:
- precede the assignment statement with the word
let
let a = n + 1
- embed the arithmetic operation inside the notation
$(())
a = $((n + 1))
$
before n in either case is allowed but not necessary.
[Example]
1 |
|
- N is now 2
[Example]
let N+=1
- Reassign N to be 1 greater, N is now 3, note that without the “let” statement, N+=1 is interpreted instead as string concatenation
let Y=$N+1
- Y is 4, the $ in front of N is not needed so this could be let Y = N + 1
let Z=Y%N
- Z is 1 (4/3 has a remainder of 1)
Y=$(($Y+1))
- Y is now 5, we can omit the $ before Y
let Y=$((Y << 2))
- Y is now 20. << is a left shift which results in a number being doubled. The number 2 in this instruction indicates that the left shift should be done twice. As Y was 5, the left shift doubles Y to 10 and then 20.
((Y++))
- Y is now 21
((Q––))
- As Q had no value, it is now −1
N=$N+1
- N has the value of 3 + 1 (literally a 3, a plus, and a 1) because
$N+1
is treated as a string concatenation operation instead of an arithmetic operation
Bash Arithmetic Operations
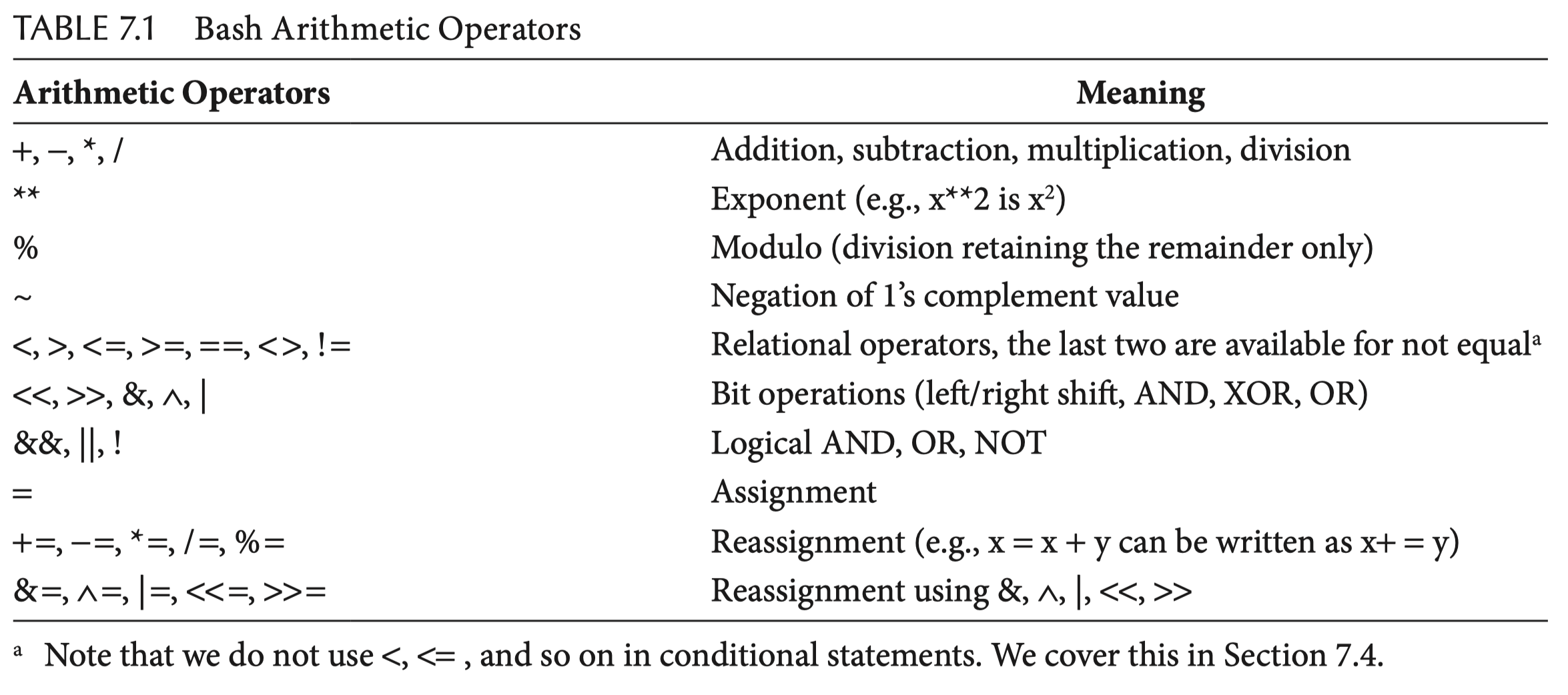
Can specify multiple assignment statements on the same line:
X = 1 Y = 2 Z = 3
Command expr
:
- performs and returns the result of a string or arithmetic operation;
- print to the terminal window.
[Example]
N=3
expr $((N+1))
- This returns 4 without changing N
expr $N+1
- This is treated as string concatenation and returns the string 3 + 1
X=‘expr $((N+1))‘
- The expr command computes and returns 4, which is then stored in X, so X stores 4 when this instruction concludes
3.5 String Operations Using expr
1 |
|
command
: substr
, index
, length
;
substr
- a substring operation;
1 |
|
string
: the string;index
: an integer indicating the position in the string to start the substring (base-1 index);length
: an integer indicating the length of the substring
expr substr abcdefg 3 2
: return the string cd
.
index
- Given two strings and it returns the location within the first string of the first character in the second string found;
1 |
|
- The index returned is the location in the master string (
str1
) of the first matching character of the search string (str2
);
expr index abcdefg qcd
: returns 3
length
- returns the length of a string
1 |
|
expr length abcdefg
: return 7
- only work if it is executed in an
expr
command. - these can be used as the right hand side of an assignment statement in which case the entire expr must be placed inside ` ` marks or inside the notation
$()
.
[Example]
PHRASE stores “hello world”
X=‘expr substr "$PHRASE" 4 6‘
- X would store “lo wor”
X=‘expr index "$PHRASE" nopq‘
- X would store 5
X=‘expr length "$PHRASE"‘
- X would store 11
3.6 Parameters
- Specified by the user at the time that the script is invoked.
- Parameters are listed on the command line after the name of the script.
./scriptexample.sh 2 3
- 2, 3 are parameters;
- they are not variables. They cannot change value while the script is executing;
- They are accessed using the notation
$n
wheren
is the index of the parameter
[Example]
./scriptexample.sh
:
1 |
|
Run:
1 |
|
Output:
1 |
|
4 Input and Output
4.1 Output with echo
output statement: echo
[Example]
1 |
|
Accept escape characters:
–e
option: echo to interpret these characters;
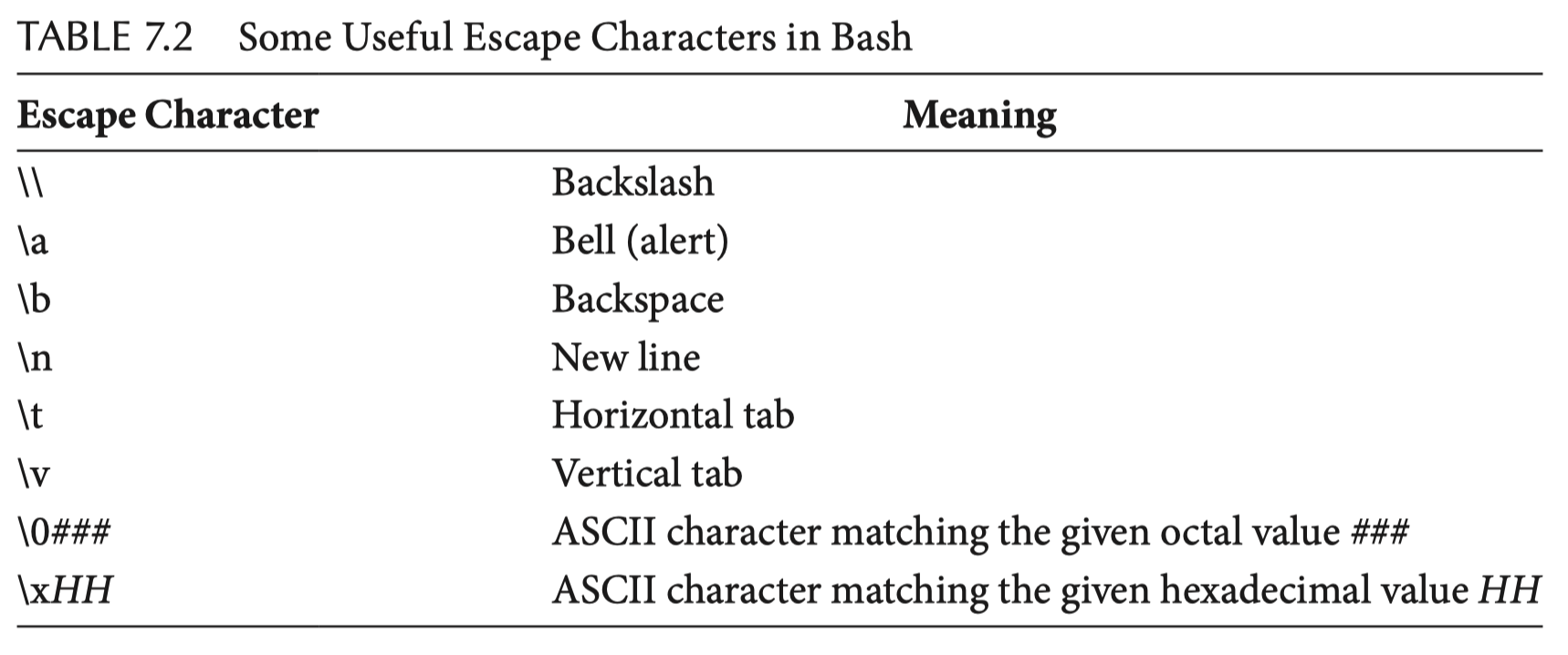
[Example]
echo –e "Hello World!\nHow are you today?"
- Hello World
- How are you today?
echo –e "January\tFebruary\tMarch\tApril"
- January February March April
echo –e "\x40\x65\x6C\x6C\x6F"
- Hello
echo –e Hello\\World
- Hello\World (recall that the quote marks are not needed for
\\
)
- The use of “” and ‘’ in echo statements is slightly different from their use in assignment statement;
- The “” return values of variables and Linux commands whereas in ‘’, everything is treated literally.
[Example]
echo $FIRST $LAST
- Frank Zappa
echo "$FIRST $LAST"
- Frank Zappa
echo '$FIRST $LAST'
$FIRST $LAST
echo "$FIRST \n$LAST"
- Frank \nZappa
echo –e $FIRST \n$LAST
- Frank nZappa
echo –e "$FIRST \n$LAST"
- Frank
- Zappa
echo –e "abc\bdef"
- abdef − the \b backs up over the ‘c’
echo –e "\0106\0162\0141\0156\0153"
- Frank
echo –e "$FIRST\t$LAST"
- Frank Zappa
4.2 Input with read
input statement: read
- The read statement is then followed by one or more variables;
- If multiple variables are specified they must be separated by spaces;
- The variable names are listed without
$
preceding them; - The read can be used to input any number of variable values.
- If the user does NOT provide the number of values as expected, any remaining variables are given NULL values.
[Example]
scriptexample.sh
:
1 |
|
Output:
1 |
|
Input (and press Enter
):
1 |
|
Output:
1 |
|
–n
option for echo
:
- causes the output to not conclude with a new line
1 |
|
Output:
1 |
|
-p
option for read
:
- outputs a prompting message prior to the input
read –p "message" var1 var2 ...
[Example]
1 |
|
Output:
1 |
|
–N
option for read
:
- Specify an integer number;
- Accept only N number of inputs;
- read
–N 10
will only read up to the first 10 characters;
- read
- The –N option will only store the input into one variable, so you would not use it if your input was for multiple variables.
–t
option for read
:
- Specify a number of seconds.
- If the input is not received from keyboard within that number of seconds, the instruction times out and no value is stored in the variable
-s
option for read
:
- Read statement execute in silent mode;
- Any input characters are not echoed to the terminal window;
- Used when inputting a password or other sensitive information;
- If read with no variables, the Bash interpreter uses the environment variable
REPLY
to store the input in to.
References
R. Fox, Linux with operating system concepts. Boca Raton, Fl: Crc Press, 2015.
Outro
尾巴
有关Linux的学习,马上继续更新。
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

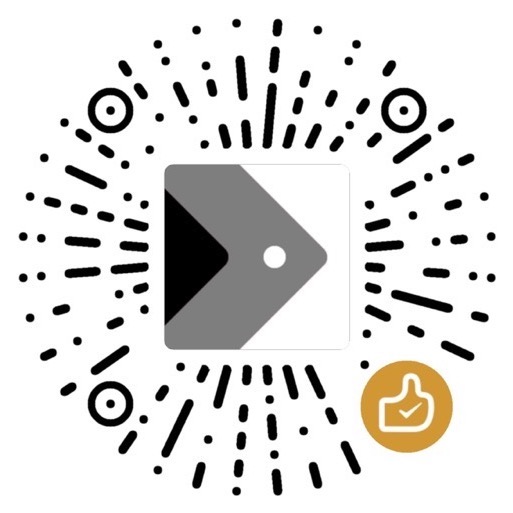