多米诺配对问题 Domino Matching with Python
Made by Mike_Zhang
数据结构与算法主题:
1. Preview
Domino Matching(多米诺配对) 问题来自于我的一门名为 Computational Thinking and Problem Solving (计算思维和问题解决 ) 的课,主要培养我们的计算思维。
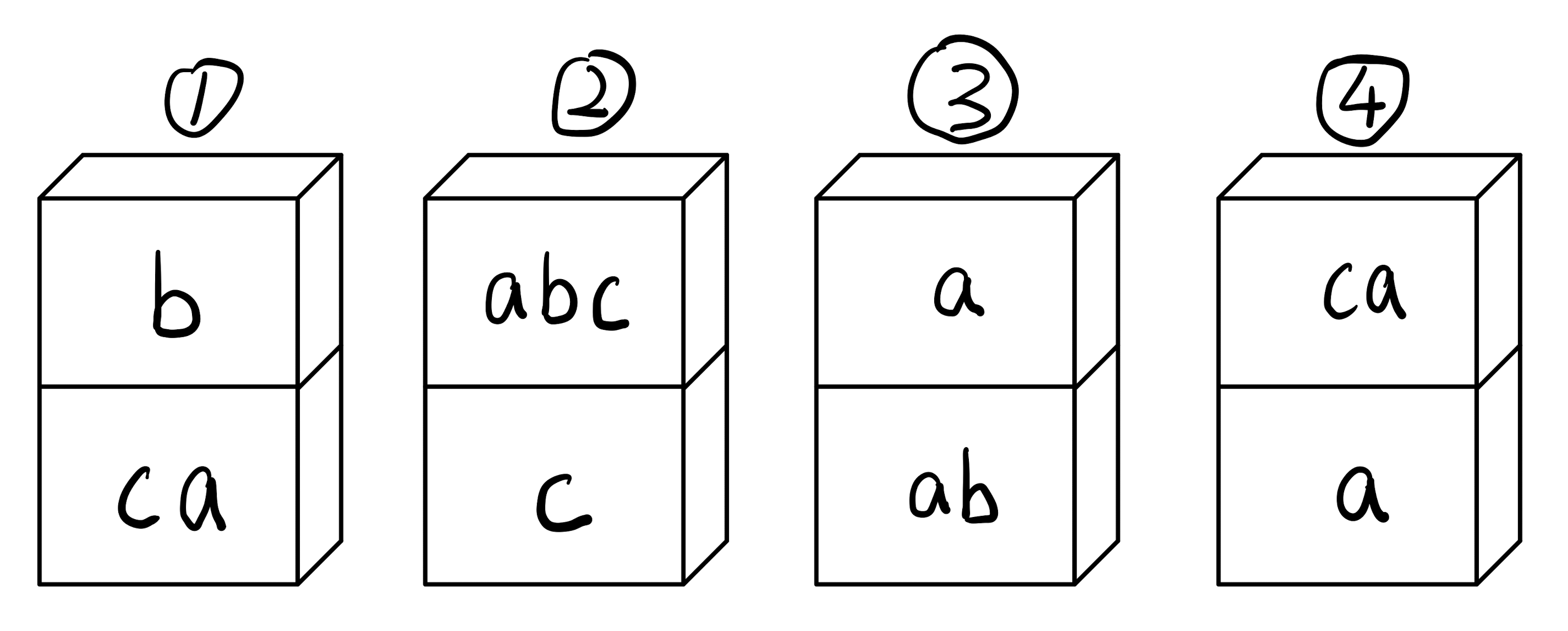
情景:
如上图所示,有四块多米诺骨牌(1~4),每块都有上和下两部分组成,上下两部分的成分是相同的(同为字母、同为数字…),每块多米诺骨牌都存在无数块。
问题:
从提供的一组多米诺骨牌(如上图中的4块)中选取多米诺骨牌,并将它们水平(如麻将般)组合起来,可重复选取,要求最后的组合体的上面部分和下面部分相同。问,此组多米诺骨牌能否组成要求的组合体,若能,组合的顺序如何?
示例:
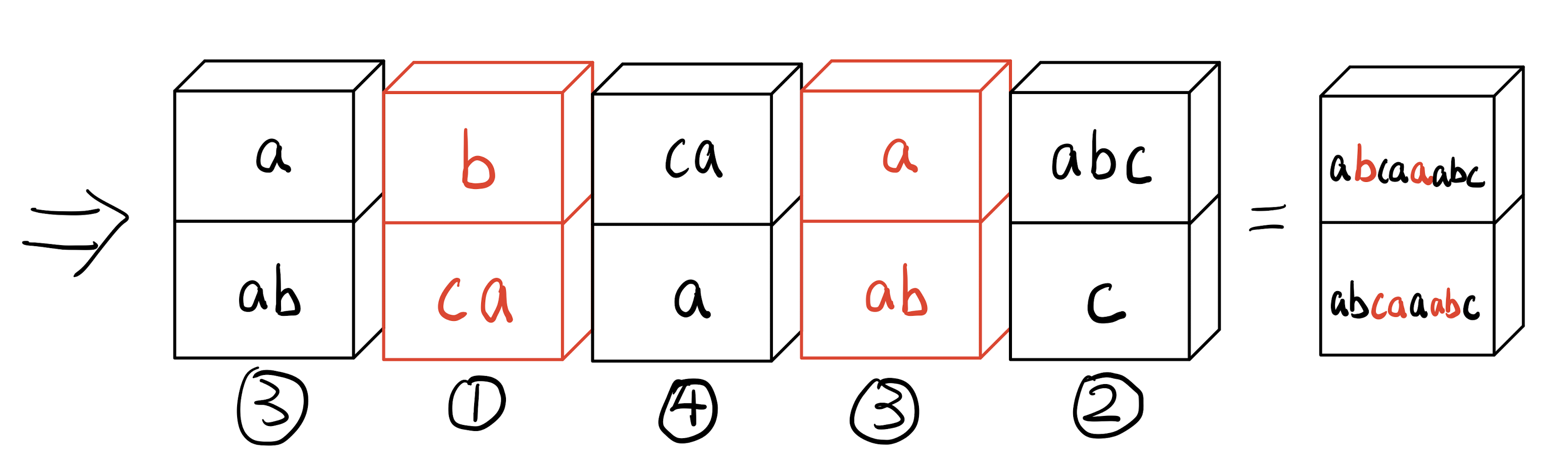
示例:
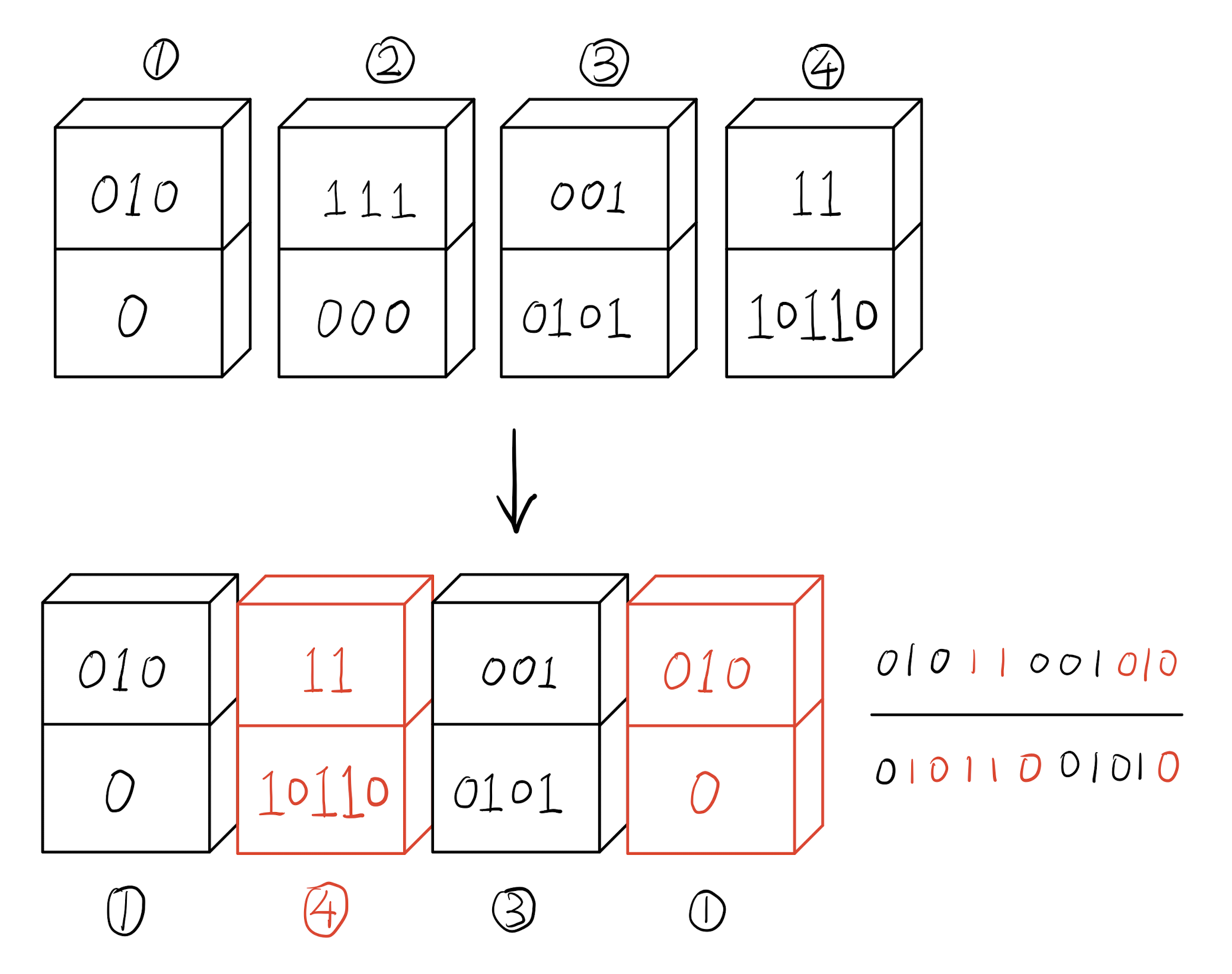
2. Computational Thinking
我们可能会对每一种组合进行尝试,但在此过程中会有一些影响组合决定的条件。
2.1 Two Conditions
当尝试把下一个多米诺骨牌连接到现有的组合体时,即将产生的新组合体会有两个检查条件:
- 其是否为完全配对体(Matched Domino),也就是最后我们希望得到的配对体。
- 其是否为部分配对体(Partially Matched Domino),检查将其添加到组合体后,此时的组合体能否有成为配对体的可能。
例如:
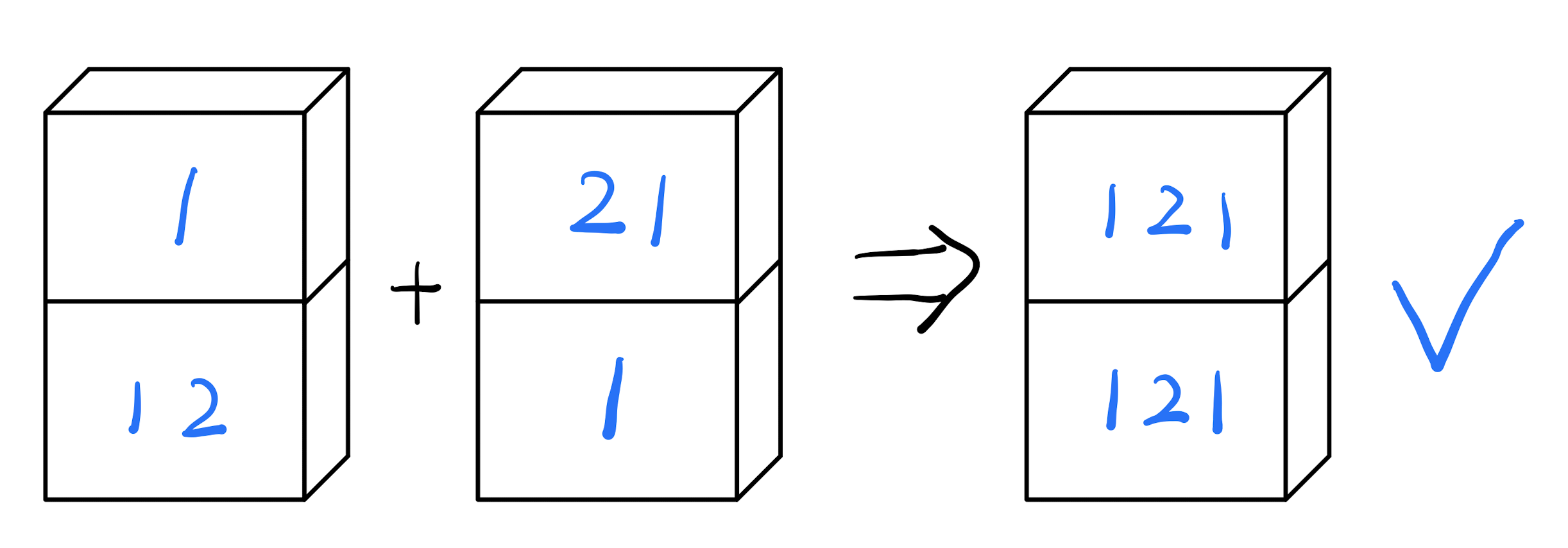
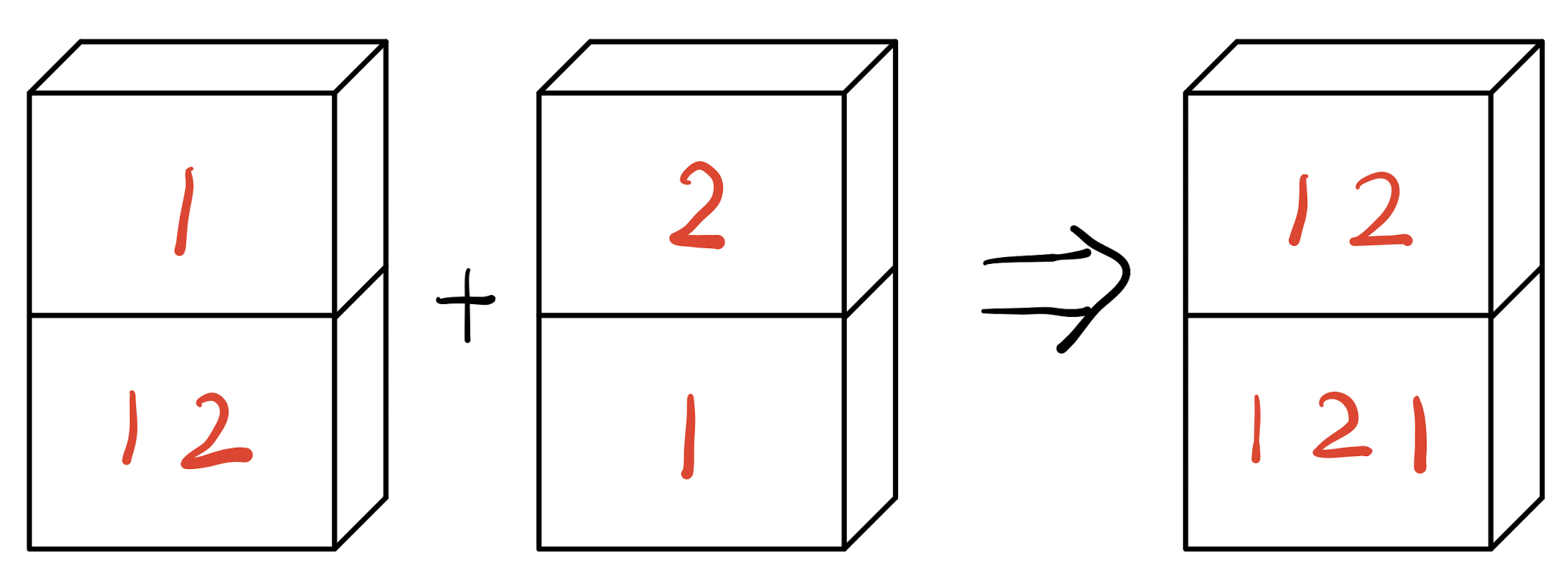
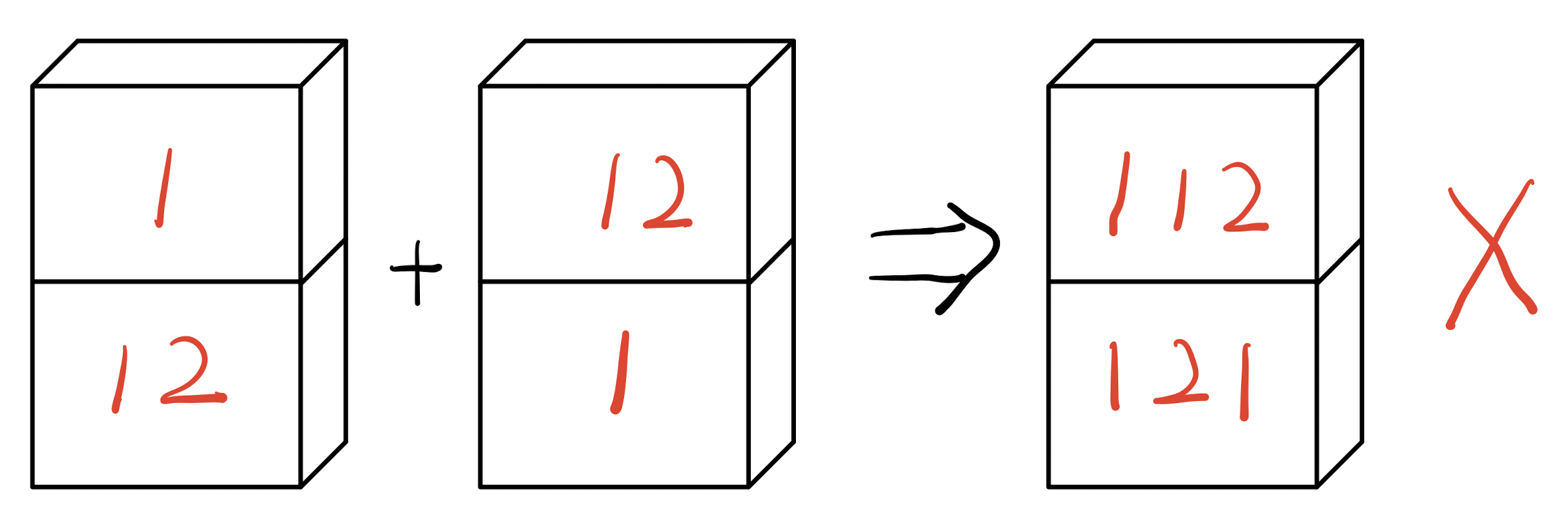
(非完全配对体 Non-Matched Domino)
在第一个例子中,若将[21,1]多米诺骨牌连接到原有组合体后,使原有组合体变成完全配对体 (Matched Domino),因此[21,1]多米诺骨牌为下一个连接的选择,并且其为最后一块多米诺骨牌。
在第二个例子中,若将[2,1]多米诺骨牌连接到原有组合体后,会使原有组合体变成部分配对体 (Partially Matched Domino),因此[2,1]多米诺骨牌可以作为下一个连接的选择。
在第三个例子中,若将[12,1]多米诺骨牌连接到原有组合体后,会使原有组合体变成非部分配对体 (Non-Partially Matched Domino),因此[12,1]多米诺骨牌不能作为下一个连接的选择。
通过这两个检查条件,可以判断哪些多米诺骨牌可以成为下一个连接的选择。
2.2 Starting Domino
万事开头难。
上面明确了选择多米诺骨牌的中间过程,但是还需要确定第一块多米诺骨牌的选择。
可以明确的是,最后的组合体上下两部分一定是相同的。因此,第一块多米诺骨牌的上下两部分肯定是部分相同的。也就是说第一块多米诺骨牌的“组合体”肯定是部分配对体 (Partially Matched Domino)。
例如:
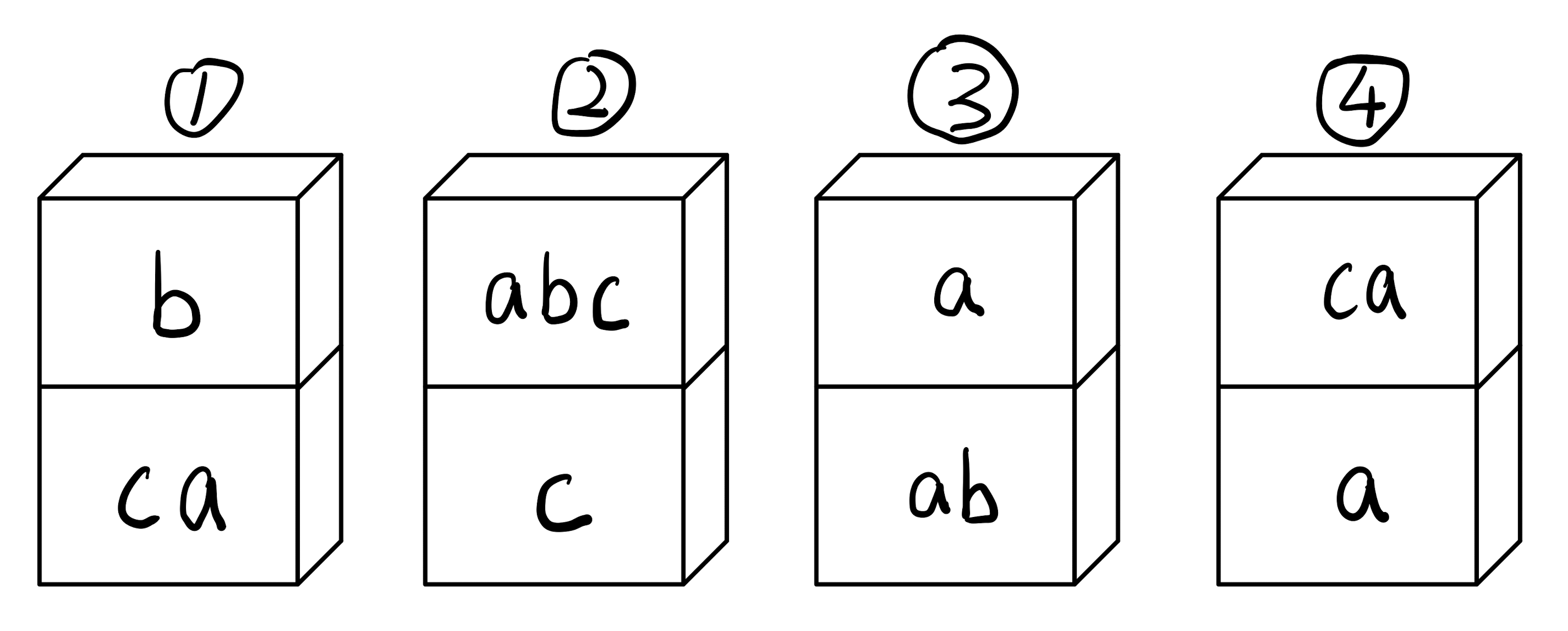
上图所示的一组多米诺骨牌中,只有3号是部分配对体 (Partially Matched Domino),因此只有其可以作为第一块多米诺骨牌。
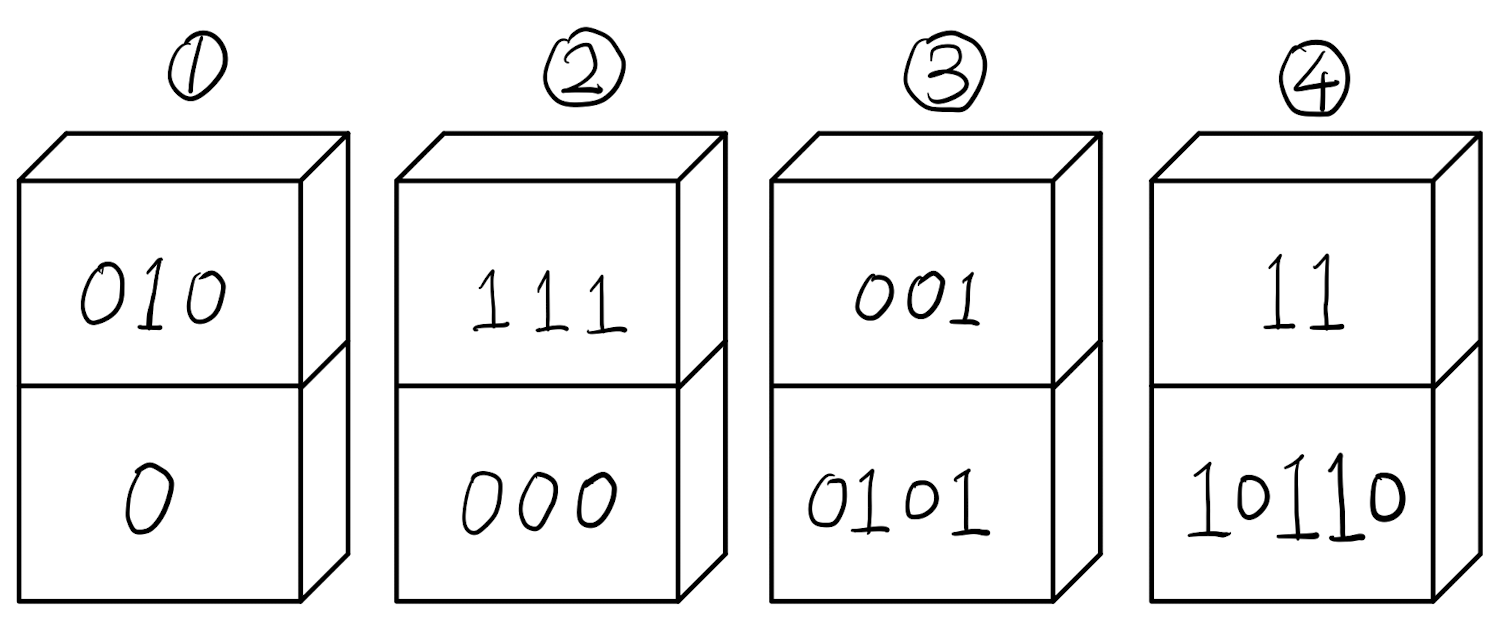
上图所示的一组多米诺骨牌中,只有1号是部分配对体 (Partially Matched Domino),因此只有其可以作为第一块多米诺骨牌。
4.3 Process
根据以上思考,可以产生对此问题的处理步骤:
- 找到第一块多米诺骨牌;
- 若找不到能作为第一块的多米诺骨牌,则这一组多米诺骨牌不能组成组合体,程序结束;
- 向现有组合体连接任意一块多米诺骨牌;
- 若其可以组成完全配对体 (Matched Domino),则完成配对,得到最后的组合体,程序结束;
- 若其可以组成部分配对体 (Partially Matched Domino),则重复步骤2;
- 若其可以组成非部分配对体 (Non-Partially Matched Domino),则将其移出现有组合体,并重复步骤2,尝试不同的多米诺骨牌;
- 若任何一块多米诺骨牌都不能连接到第一块多米诺骨牌后,则程序结束。
3. Problem Solving with Python Code
以下为多米诺配对问题 (Domino Matching) 的 Python 解决方法。
1 |
|
写在最后
解决多米诺配对问题的方法有很多种,我的方法并非最优解,欢迎大家尝试。
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

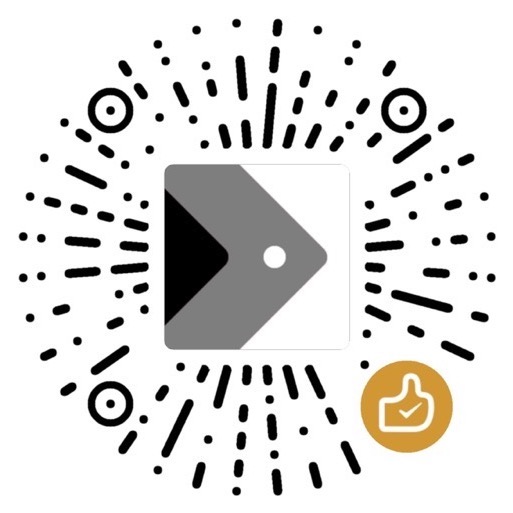