C Learning 1 - Data Type & IO
Made by Mike_Zhang
Unfold C/C++ Topics | 展开 C/C++ 主题 >
1. Introduction
1 |
|
2. Basic Data Type
2.1 Integer
1 |
|
2 byte for 16-bit machine, 4 byte for 32-bit machine
Type | Size | Range |
---|---|---|
(signed) int |
2 or 4 bytes | -32,768 ~ 32,767 or -2,147,483,648 ~ 2,147,483,647 |
unsigned int / unsigned |
2 or 4 bytes | 0 ~ 65,535 or 0 ~ 4,294,967,295 |
(signed) short int / short |
2 bytes | -32,768 ~ 32,767 |
unsigned short int / unsigned short |
2 bytes | 0 ~ 65,535 |
(signed) long int / long |
4 bytes | -2,147,483,648 ~ 2,147,483,647 |
unsigned long int / unsigned long |
4 bytes | 0 ~ 4,294,967,295 |
long long int / long long |
8 bytes | -9,223,372,036,854,775,808 ~ 9,223,372,036,854,775,807 |
2.2 Real Floating Point
1 |
|
Type | Size | Range | Precision |
---|---|---|---|
float |
4 bytes | 1.2E-38 ~ 3.4E+38 | 6 decimal digits |
double |
8 bytes | 2.3E-308 ~ 1.7E+308 | 15 decimal digits |
long double |
10 bytes | 3.4E-4932 ~ 1.1E+4932 | 19 decimal digits |
2.3 Characters
1 |
|
Type | Size | Range |
---|---|---|
char |
1 byte | -128 ~ 127 or 0 ~ 255 |
unsigned char |
1 byte | 0 ~ 255 |
signed char |
1 byte | -128 ~ 127 |
char
type actually stores integers, not characters, e,g, ASCII code;- A single character contained between single quotes is a C character constant, can NOT between double quotes, which is a String;
- Can also use the numerical code to assign values.
- Attempting to assign
'ABC'
to a char variable results in only the last 8 bits being used, so the variable gets the value ‘C’.
2.4 Escape Sequences
Nonprinting Characters
Sequence | Meaning |
---|---|
\a |
Alert (beep) |
\b |
Backspace |
\f |
Form feed |
\n |
Newline |
\r |
Carriage return |
\t |
Horizontal tab |
\v |
Vertical tab |
\\ |
Backslash (\) |
\' |
Single quote (\’) |
\" |
Double quote (\”) |
\? |
Question mark (?) |
\0oo |
Octal value. (o represents an octal digit.) |
\xhh |
Hexadecimal value. (h represents a hexadecimal digit.) |
Examples of Integer Constants
type | hexadecimal | octal | decimal |
---|---|---|---|
char | \0x41 | \0101 | N.A. |
int | 0x41 | 0101 | 65 |
unsigned int | 0x41u | 0101u | 65u |
long | 0x41L | 0101L | 65L |
unsigned long | 0x41UL | 0101UL | 65UL |
long long | 0x41LL | 0101LL | 65LL |
unsigned long long | 0x41ULL | 0101ULL | 65ULL |
2.5 Boolean
1 |
|
Type | Size | Range |
---|---|---|
_Bool |
1 byte | 0: false, non-zero: true |
- A
_Bool
variable can only have a value of 1 (true) or 0 (false); - C considers any non-zero value to be true;
- the
_Bool
type really is just an integer type (unsigned integer);
3. Character Strings
A character string is an array of one or more characters with \0
as the terminating null character to mark the end of a string.
H | e | l | l | o | , | W | o | r | l | d | ! | \0 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
3 ways of declaration initialization of a string:
- Specify an array size large enough to hold the string with double quotation marks.
1 |
|
- Standard array initialization form, with the last element set to
\0
.
1 |
|
- Omit the size in an initializing declaration.
1 |
|
Basic string functions
Function | Description | Example |
---|---|---|
strlen(str) |
This function returns the number of characters, NOT including the terminating null character, found in the string s. | char str[20] = "Hello, World!"; strlen(str); // 13 |
strcat(s1, s2) |
The string pointed to by s2 is copied to the end of the string pointed to by s1 . The first character of the s2 string is copied over the null character of the s1 string. The return value is s1 . |
char s1[20] = "Hello, "; char s2[20] = "World!"; strcat(s1, s2); // s1 = "Hello, World!" |
strcmp(s1, s2) |
This function returns a positive value if the s1 string follows the s2 string in the machine collating sequence, the value 0 if the two strings are identical, and a negative value if the first string precedes the second string in the machine collating sequence. |
char s1[]= "A"; char s2[] = "B"; strcmp(s1, s2); // -1 |
strcpy(s1, s2) |
This function copies the string (including the null character) pointed to by s2 to the location pointed to by s1 , instead of address. The return value is s1 . |
char s1[20]; char s2[] = "Hello, World!"; strcpy(s1, s2); // s1 = "Hello, World!" |
strrchr(s, c) |
This function returns a pointer to the last occurrence of the character c in the string s . (The terminating null character is part of the string, so it can be searched for.) The function returns the null pointer if the character is not found. |
char str[] = "Hello, World!"; strrchr(str, 'o'); // "orld!" |
strstr(s1, s2) |
This function returns a pointer to the first occurrence of string s2 in string s1 . The function returns the null pointer if the string is not found. |
char str[] = "Hello, World!"; strstr(str, "World"); // "World!" |
4. Constants and Preprocessor
Two ways of defining constants:
#define
preprocessor
1 |
|
- It is a compile-time substitution.
- When program is compiled, the value $10$ will be substituted everywhere you have used
VAL
.
- When program is compiled, the value $10$ will be substituted everywhere you have used
const
keyword
1 |
|
- Using the const keyword to convert a declaration for a variable into a declaration for a constant.
- This makes
VAL
into a read-only value. That is, you can displayVAL
and use it in calculations, but you cannot alter the value ofVAL
.
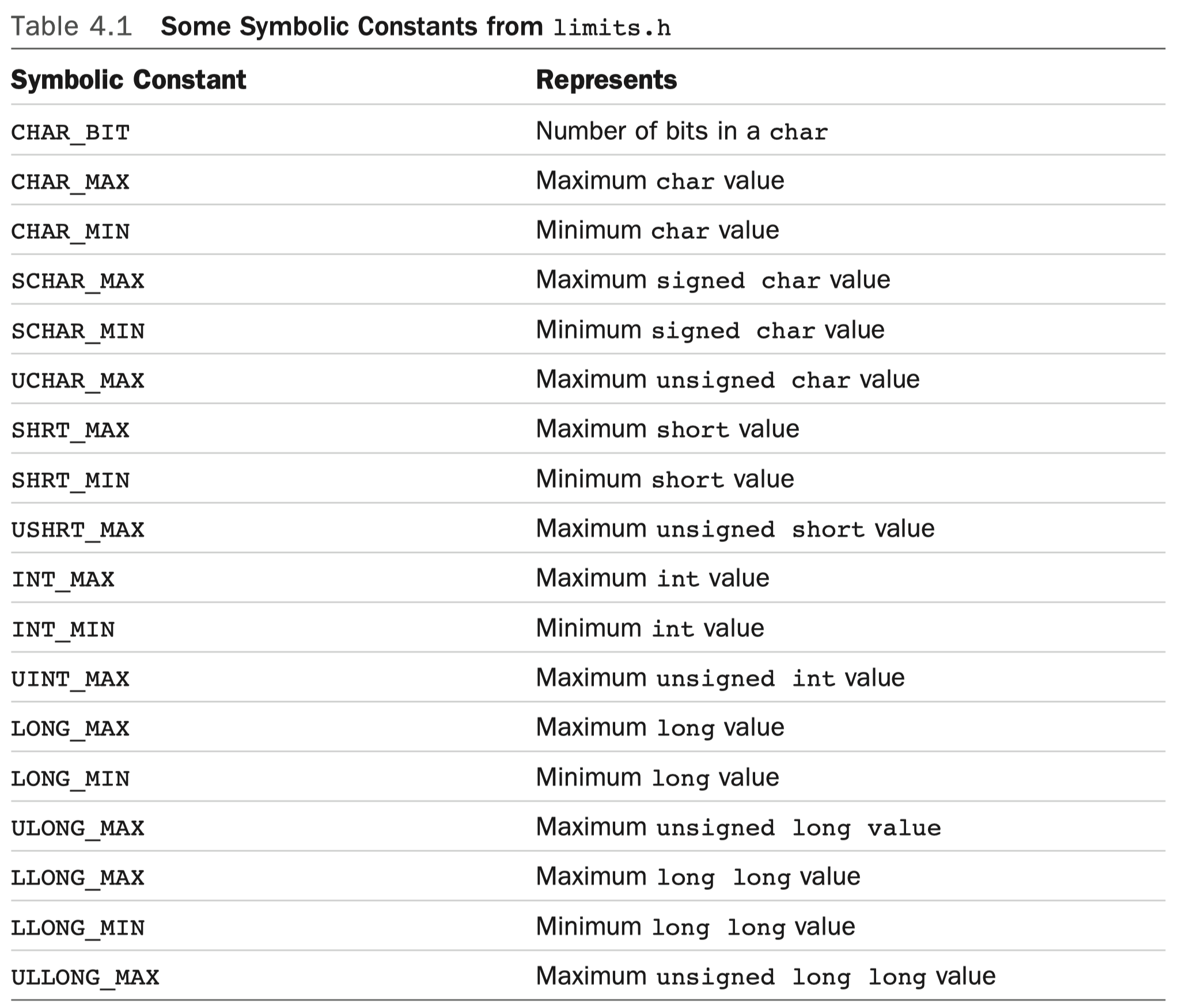
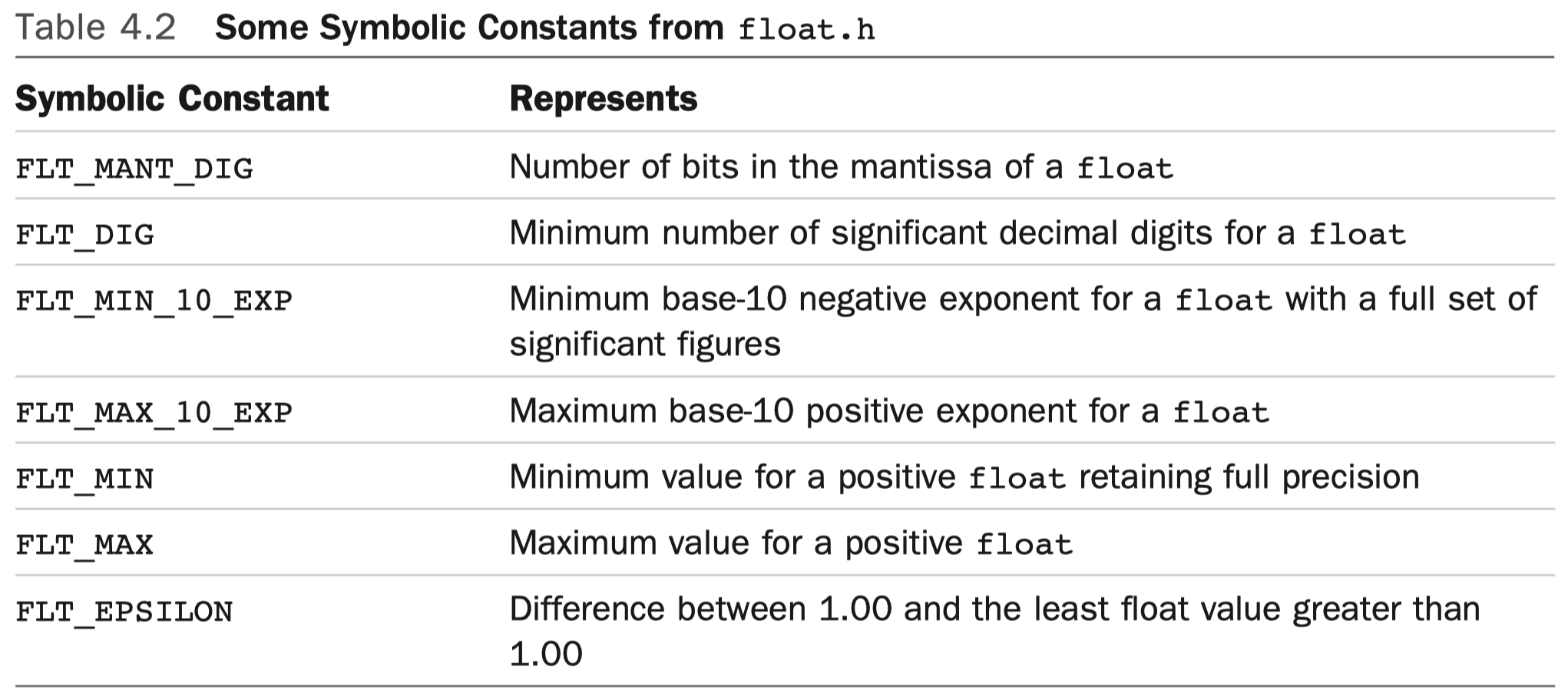
5 I/O
5.1 printf
and scanf
5.1.1 printf
Print a variable depend on the variable type.
1 |
|
Arguments for printf()
:

Anatomy of a control statement:
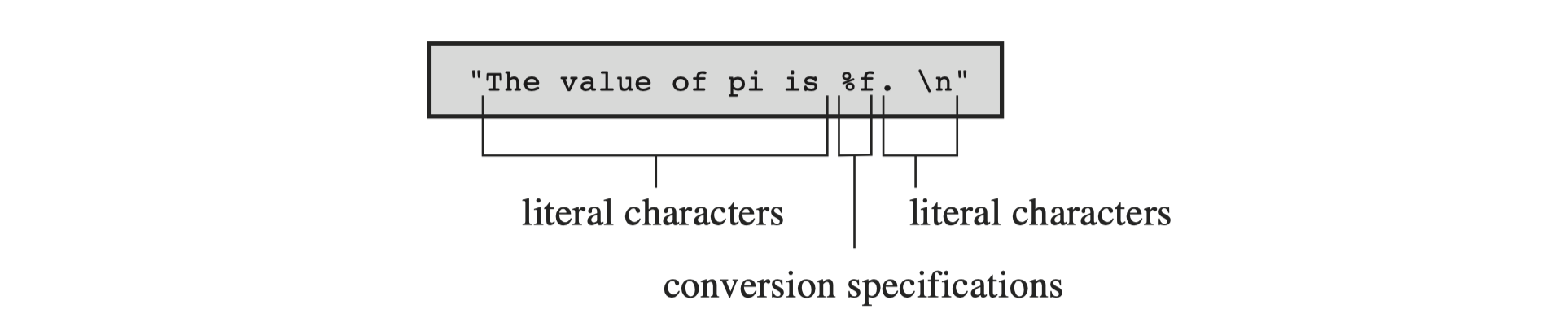
- Conversion specifications:
- specify how the data is to be converted into displayable form.
[Example]
1 |
|
Output:
1 |
|
Conversion Specifiers and the Resulting Printed Output
Conversion | Resulting Printed Output |
---|---|
%a |
Floating-point number, hexadecimal digits and p-notation (C99/C11). |
%A |
Floating-point number, hexadecimal digits and P-notation (C99/C11). |
%c |
Single character. |
%d |
Signed decimal integer. |
%e |
Floating-point number, e-notation. |
%E |
Floating-point number, e-notation. |
%f |
Floating-point number, decimal notation. |
%g |
Use %f or %e, depending on the value. The %e style is used if the exponent is less than −4 or greater than or equal to the precision. |
%G |
Use %f or %E, depending on the value. The %E style is used if the exponent is less than −4 or greater than or equal to the precision. |
%i |
Signed decimal integer (same as %d). |
%o |
Unsigned octal integer. |
%p |
A pointer. |
%s |
Character string. |
%u |
Unsigned decimal integer. |
%x |
Unsigned hexadecimal integer, using hex digits 0f. |
%X |
Unsigned hexadecimal integer, using hex digits 0F. |
%% |
Prints a percent sign. |
Conversion Specification Modifiers
Modifier | Meaning | Example |
---|---|---|
flag |
Five flags (-, +, space, #, and 0). Zero or more flags may be present. | “%-10d”. |
digit(s) |
The minimum field width. A wider field will be used if the printed number or string won’t fit in the field. | “%4d”. |
.digit(s) |
Precision. For %e, %E, and %f conversions, the number of digits to be printed to the right of the decimal. For %g and %G conversions, the maximum number of significant digits. For %s conversions, the maximum number of characters to be printed. For integer conversions, the minimum number of digits to appear; leading zeros are used if necessary to meet this minimum. Using only . implies a following zero, so %.f is the same as %.0f. | “%5.2f” prints a float in a field five characters wide with two digits after the decimal point. |
h |
Used with an integer conversion specifier to indicate a short int or unsigned short int value. | “%hu”, “%hx”, and “%6.4hd”. |
hh |
Used with an integer conversion specifier to indicate a signed char or unsigned char value. | “%hhu”, “%hhx”, and “%6.4hhd”. |
j |
Used with an integer conversion specifier to indicate an intmax_t or uintmax_t value; these are types defined in stdint.h. | “%jd” and “%8jX”. |
l |
Used with an integer conversion specifier to indicate a long int or unsigned long int. | “%ld” and “%8lu”. |
ll |
Used with an integer conversion specifier to indicate a long long int or unsigned long long int. (C99). | “%lld” and “%8llu”. |
L |
Used with a floating-point conversion specifier to indicate a long double value. | “%Lf” and “%10.4Le”. |
t |
Used with an integer conversion specifier to indicate a ptrdiff_t value. This is the type corresponding to the difference between two pointers. (C99). | “%td” and “%12ti”. |
z |
Used with an integer conversion specifier to indicate a size_t value. This is the type returned by sizeof. (C99). | “%zd” and “%12zx”. |
Five flags
Flag | Meaning | Example |
---|---|---|
+ |
Signed values are displayed with a plus sign, if positive, and with a minus sign, if negative. | “%+6.2f”. |
space |
Signed values are displayed with a leading space (but no sign) if positive and with a minus sign if negative. A + flag overrides a space. | “% 6.2f”. |
# |
Use an alternative form for the conversion specification. Produces an initial 0 for the %o form and an initial 0x or 0X for the %x or %X form, respectively. For all floating-point forms, # guarantees that a decimal-point character is printed, even if no digits follow. For %g and %G forms, it prevents trailing zeros from being removed. | “%#o”, “%#8.0f”, and “%+#10.3E”. |
0 |
For numeric forms, pad the field width with leading zeros instead of with spaces. This flag is ignored if a - flag is present or if, for an integer form, a precision is specified. | “%010d” and “%08.3f”. |
[Example]
1 |
|
Output:
1 |
|
5.1.2 scanf
It converts string input into various forms: integers, floating-point numbers, characters, and C strings.
The format of scanf
is similar to printf
:
1 |
|
- The scanf() function uses whitespace (newlines, tabs, and spaces) to decide how to divide the input into separate fields.
- It matches up consecutive conversion specifications to consecutive fields, skipping over the whitespace in between.
Two rules of using pointers to variables.
- Use
scanf()
to read a value for one of the basic variable types, precede the variable name with an&
. - Use
scanf()
to read a string into a character array, don’t use an&
.
1 |
|
Output:
1 |
|
Conversion Specifiers for scanf()

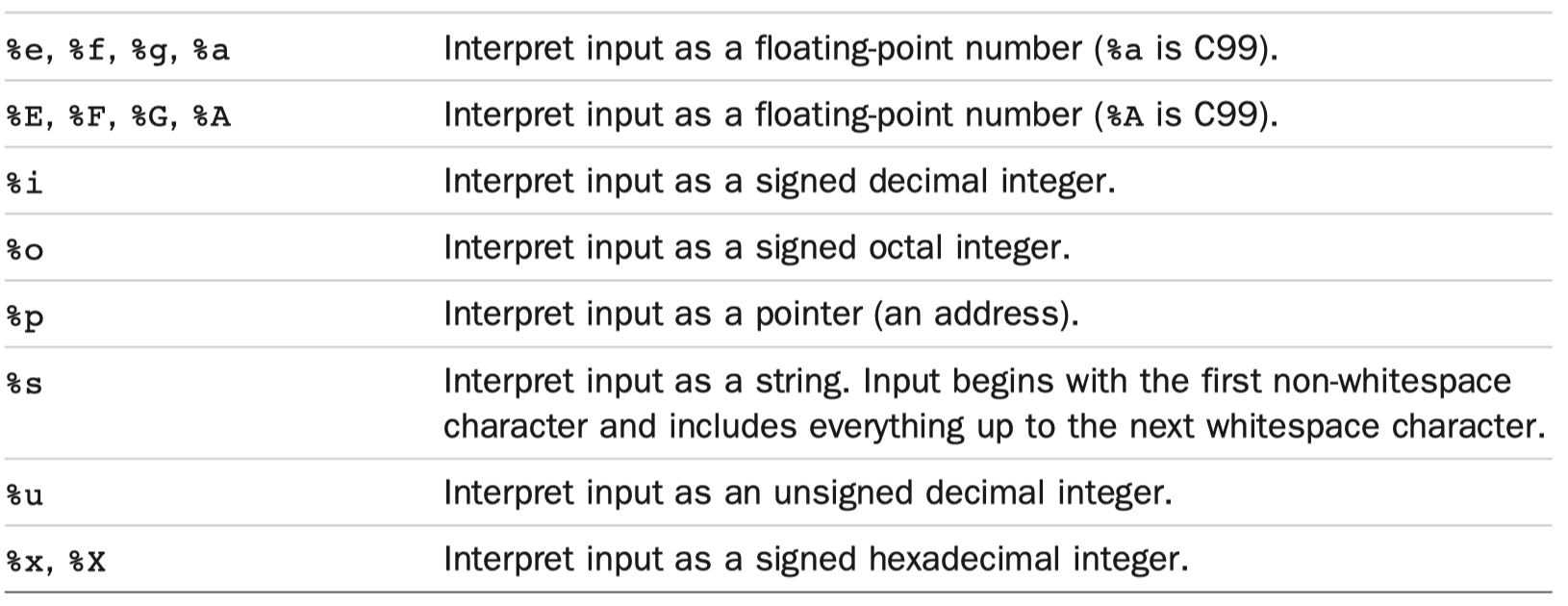
Conversion Modifiers for scanf()
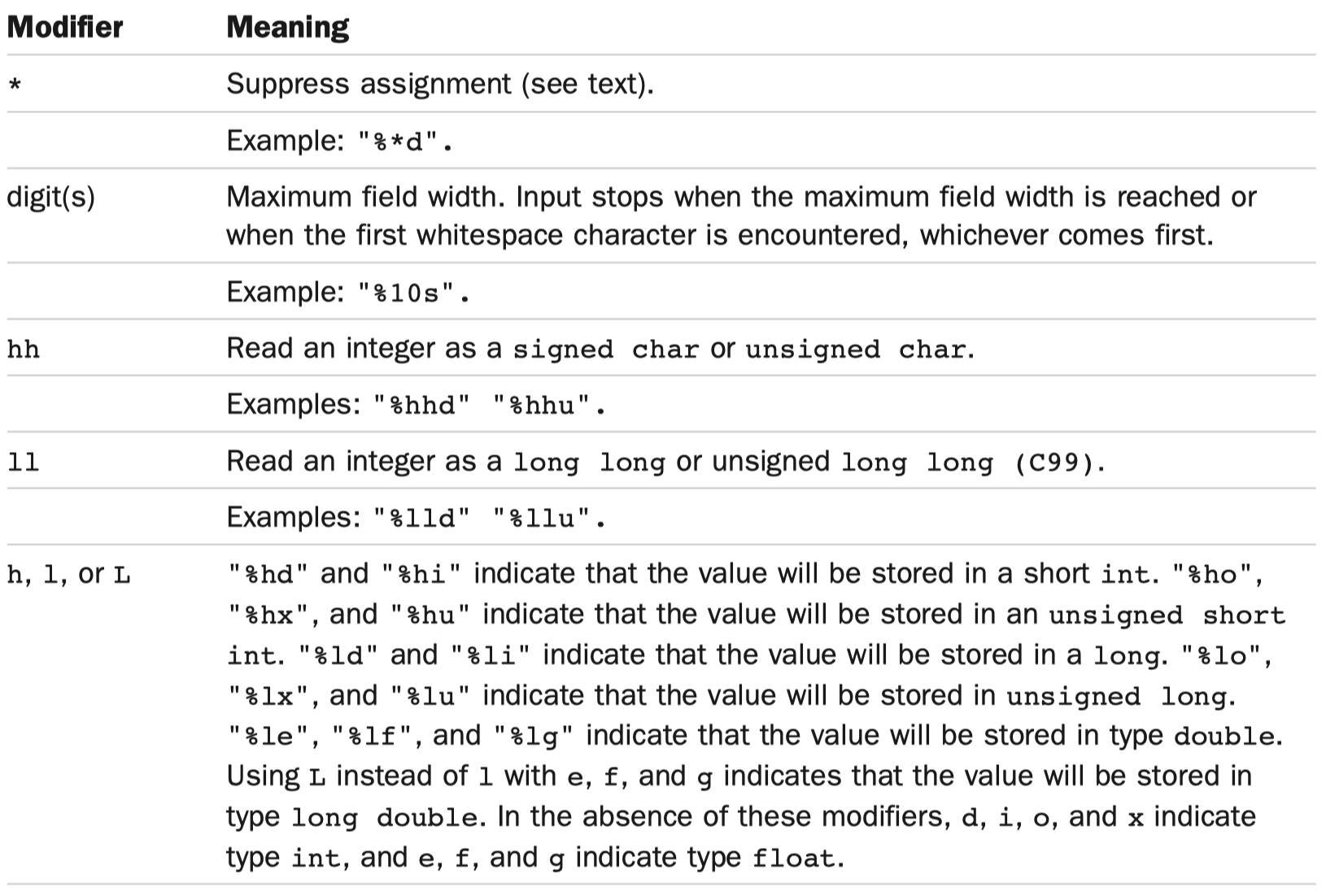
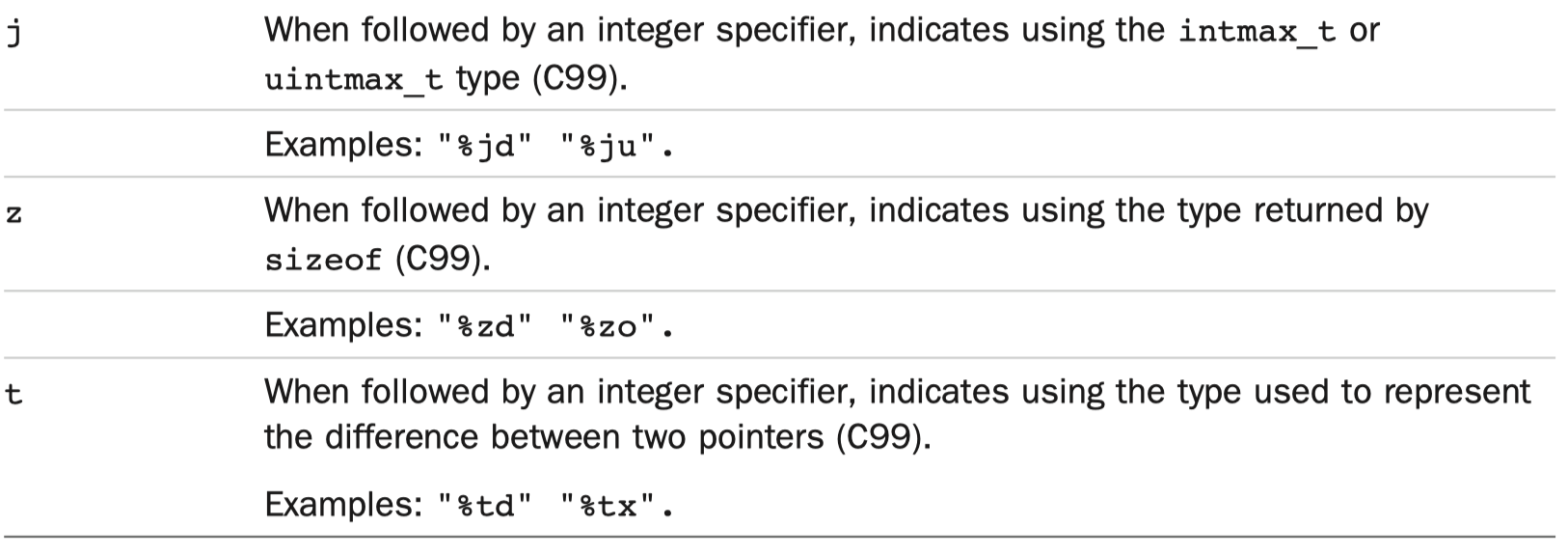
5.2 getchar
and putchar
int getchar(void);
Reads the next character from
stdin
buffer.
Return value
- The obtained character on success or EOF on failure.
- If the failure has been caused by end-of-file condition, additionally sets the eof indicator (see feof()) on stdin. If the failure has been caused by some other error, sets the error indicator (see ferror()) on stdin.
If the buffer is empty: ask for user input, all the input characters are stored in the buffer;
- If the buffer is not empty: read the first character in the buffer, no need to ask for user input.
int putchar(int ch);
Writes a character ch to
stdout
. Internally, the character is converted to unsigned char just before being written.
- Parameters
ch
- character to be written
- Return value
- On success, returns the written character.
- On failure, returns EOF and sets the error indicator (see ferror()) on stdout.
Classical example:
This process is called echoing the input. It uses a while loop that terminates when the # character is encountered.
1 |
|
Ouptut:
1 |
|
5.3 gets
and puts
char* gets(char* str);
Reads
stdin
into given character string until a newline character is found or end-of-file occurs.
- Parameters
str
- character string to be written
- Return value
- str on success, a null pointer on failure.
- If the failure has been caused by end of file condition, additionally sets the eof indicator (see std::feof()) on stdin. If the failure has been caused by some other error, sets the error indicator (see std::ferror()) on stdin.
- str on success, a null pointer on failure.
int puts(const char *str);
Writes every character from the null-terminated string str and one additional newline character ‘\n’ to the output stream stdout, as if by repeatedly executing fputc.
The terminating null character from str is not written.
- Parameters
str
- character string to be written
- Return value
- On success, returns a non-negative value
- On failure, returns EOF and sets the error indicator (see ferror()) on stream.
1 |
|
Output:
1 |
|
References
S. Prata, C primer plus. Upper Saddle, Nj: Addison-Wesley, 2014.
原创文章,转载请标明出处
Made by Mike_Zhang

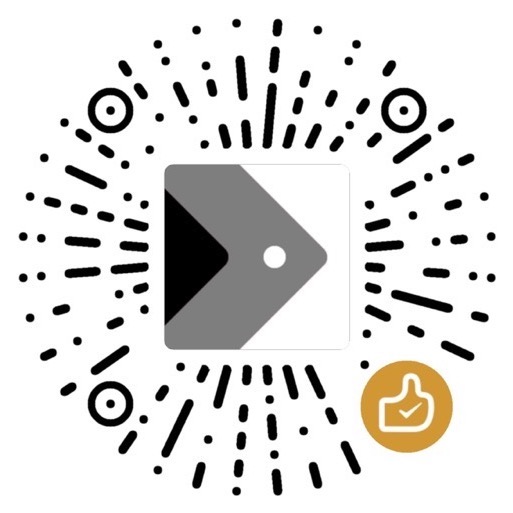