Java基础学习2-表达式、控制流与I/O
Made by Mike_Zhang
Java主题:
本文将记录Java基础学习的三大内容 - 表达式 (Expressions) 、控制流 (Control Flow) 与 I/O。
1. 表达式 (Expressions)
1.1 转义字符 (Escape Character)
转义字符 (Escape Character) | 描述 | 解释 |
---|---|---|
‘\n’ | new line | 换行-光标移动至下一行开头 |
‘\t’ | tab | 水平移动-光标移动至下一个TAB后 |
‘\b’ | backspace | 退格(删除)-光标移动至前一列 |
‘\r’ | return | 回车-光标移动至本行开头 |
‘\f’ | form feed | 换页-光标移动至下页开头 |
‘\\\’ | backslash | |
‘\‘’ | single quote | |
‘\“‘ | double quote | |
‘\0’ | null | |
‘\000’ | 1-3位八进制数 | 范围\000~\377 |
‘\u0000’ | Unicode字符 4位16进制数 (‘\u0000’为空字符) | 范围0~65535 |
运行以下代码:
1 |
|
输出:
1 |
|
1.2 运算符 (Operator)
1.2.1 数字运算符 (Arithmetic Operator)
数字运算符 (Arithmetic Operator) | 描述 |
---|---|
+ | addition |
- | subtraction |
$*$ | multiplication |
/ | division |
% | modulo (take the remainder) |
1.2.2 字符串连接符 (String Concatenation)
$+$为字符串连接符 (String Concatenation),例子如下:1
2
3
4String aString = "This";
String bString = "is";
String cString = aString + bString;
Stirng dString = cStirng + "my book"; // dString = "This is my book"
1.2.3 自增与自减运算符 (Increment and Decrement Operator)
例如:1
2
3
4int i = 1;
int j = i++;
// ...
int k = i--;
类似于:1
2
3
4
5
6int i = 1;
int j = i;
i = i + 1;
// ...
int k = i;
i = i - 1;
上面的例子中,自增或自减符号位于变量后,则先进行赋值操作,再对变量进行自增或自减。若自增或自减符号位于变量前,则先对变量进行自增或自减,再进行赋值操作。如下:
1 |
|
类似于:1
2
3
4
5
6int i = 1;
i = i + 1;
int j = i;
// ...
i = i - 1;
int k = i;
1.2.4 逻辑运算符 (Logical Operator)
数字逻辑运算符 | 描述 |
---|---|
< | less than |
<= | less than or equal to |
> | greater than |
>= | greater than or equal to |
== | equal to |
!= | not equal to |
布尔逻辑运算符 | 描述 | 例子 |
---|---|---|
! | not(prefix) | !true = false |
&& | AND | true && false = false |
|| | OR | true || false = true |
^ | Exclusive OR | true ^ false = true |
1.2.5 位运算符 (Bitwise Operator)
假设:1
2byte a = (byte)0B10101010;
byte b = (byte)0B00100000;
位运算符 (Bitwise Operator) | 描述 | 例子 |
---|---|---|
~ | complement | (byte)~a = 0B01010101 |
& | AND | (byte)(a & b) = 0B00100000 |
| | OR | (byte)(a | b) = 0B10101010 |
^ | Exclusive OR | (byte)(a ^ b) = 0B10001010 |
<< | Signed left shift, fill with 0 | (bytr)(a << 2) = 0B10101000 |
>> | Signed right shift, fill with sign bit | (bytr)(a >> 2) = 0B11101010 |
>>> | Unsigned right shift, fill with 0 | (bytr)(a >> 2) = 0B00101010 |
1.2.6 赋值运算符 (Assignment Operator)
=
为赋值符号,把一个值赋值给某一变量,语法如下:
variable = expression
例子:i = 2;
以下情况会从右往左进行赋值:i = j = 3;
相当于:j = 3;
i = j;
1.2.7 复合赋值运算符 (Compound Assignment Operator)
语法如下:variable op= expression
相当于:variable = variable op expression
例如:i =+ 1;
相当于:i = i + 1
若出现下列的表达式,则需要根据运算符号优先级来判断运算顺序:
i = j += (k * 2) / 8
运算符号优先级详见1.2.9节。
1.2.8 条件表达式 (Conditional Expression)
语法如下:boolean-expression ? exp1 : exp2
例如:1
2System.out.println((num % 2 == 0)? "EVEN" : "ODD")
解释:
如果 boolean-expression == true
那么整个表达式等于exp1
,否则等于exp2
。
1.2.9 运算符优先级 (Operator Precedence)
运算符 | 描述 | 级别 | 运算方向 |
---|---|---|---|
[ ] | access array element | 1 | L to R |
. | access object member | 1 | L to R |
( ) | invoke a method | 1 | L to R |
++ | post-increment | 1 | L to R |
— | post-decrement | 1 | L to R |
++ | pre-increment | 2 | R to L |
— | pre-decrement | 2 | R to L |
+ | unary plus | 2 | R to L |
- | unary minus | 2 | R to L |
! | logical NOT | 2 | R to L |
~ | bitwise NOT | 2 | R to L |
( ) | cast | 3 | R to L |
new |
object creation | 3 | R to L |
* | multiplication | 4 | L to R |
/ | division | 4 | L to R |
% | modulo | 4 | L to R |
+ - | addition, subtraction | 5 | L to R |
+ | string concatenation | 5 | L to R |
<< >> >>> | shift | 6 | L to R |
< <= > >= instanceof |
relational type comparison | 7 | L to R |
== != | equality | 8 | L to R |
& | bitwise AND | 9 | L to R |
^ | bitwise XOR | 10 | L to R |
| | bitwise OR | 11 | L to R |
&& | conditional AND | 12 | L to R |
|| | conditional OR | 13 | L to R |
?: | conditional | 14 | R to L |
= += -= *= /= %= &= ^= |= <<= >>= >>>= | assignment | 15 | R to L |
2. 控制流 (Control Flow)
2.1 条件语句 (Conditional Statement)
2.1.1 if
表达式
语法如下:
1 |
|
1 |
|
trueBody
、falseBody
、firstBody
、secondBody
和thirdBody
可以为单行的语句或者是语句块(写在{}
内),例如:
1 |
|
2.1.2 switch
表达式
语法如下:
1 |
|
selector
会与每个value
进行比较,若相等则运行后面的statement
语句,若没有一个相等的,则运行default
之后的语句。 每个case
中的break;
语句是可选择的,一般选择加上,以便满足case
条件后直接退出,若不加,则会运行其之后的语句,直到遇到break;
语句再退出。default
语句后一般不需要break;
语句,因为此语句通常放在最后,运行default后会直接退出。但是如果default
语句后还有语句,则需要break;
语句。但是不推荐default
语句后再加语句,这违背了初衷,只有前面所有情况都不满足之后再使用default
语句。因此推荐把default
语句放在最后,此时,可选择是否加上break;
语句。
selector
应为整型数据,如char
,byte
,short
,int
,boolean
,String
或者enum
。
如需要使用非整型数据,如long
,float
或者double
,则需要使用if
语句。
2.2 循环语句 (Loop Statement)
2.2.1 while
循环
语法如下:
1 |
|
例子:
1 |
|
2.2.2 do-while
循环
语法如下:
1 |
|
例子:
1 |
|
与while
循环不同的是,每一次do-while
循环先执行循环体的语句,最后进行循环条件的判断。这种循环可以实现进入循环后再询问用户的输入,不用在循环前询问。
2.2.3 for
循环
语法如下:
1 |
|
例子:
1 |
|
for
循环可以转换为while
循环,如下:
1 |
|
2.2.4 for-each
循环
语法如下:
1 |
|
container
为elementType
类型的数组,此循环会遍历数组内的元素。例如:
1 |
|
此循环遍历arr
数组的全部元素,最后得出其总和。
注意:for-each
循环并不会访问到具体的元素及其下标,因此以下代码是不合法的:
1 |
|
所以如果要进行对具体元素的修改,则需要用for
或者while
循环,如下:1
2
3
4int[] arr = {1,2,3,4};
for (int i = 0; i < arr.length; i++){
arr[i]++;
}
2.2.5 强制跳转 (Unconditional Branching)
return
- 当一个方法的返回值被定义为
void
后,只有运行完此方法的最后一行代码之后才会跳出此方法。但是当一个方法的返回值定义到一个具体的数据类型,当运行到return
关键字后就会跳出此方法。return
语句必须是一个方法中最后被执行的语句,其后的语句都不会被执行。return
语句行不一定是方法代码的最后一行,但是最后被运行的那一行。如下:
1 |
|
break
break
语句可以跳出目前所在的循环体(e.g. for
, while
, do-while
, switch
)。跳出后开始执行此循环的后一行代码。
continue
continue
语句会跳过其后的本次正在循环的代码,并且开始执行此循环体的下一次循环(如果还满足循环的条件),并不会像break
语句一样直接跳出循环体。
3. I/O
3.1 输出 (output)
语法如下:1
2System.out.print("Hello,World!");
System.out.println("Hello,World!"); // followed by a newlineSystem.out
对象属于java.io.PrintStream
类的一个实例
3.2 输入 (input)
一般语法如下:1
2
3
4
5
6
7
8import java.util.Scanner;
public class Example{
public static void main(String[] args){
Scanner input = new Scanner(System.in);
System.out.print("Enter :");
double num = input.nextDouble();
}
}
Scanner
会根据分隔符把输入内容分成好几个小节后再进行一一读取。
分隔符一般为whitespace(i.e. space, tabs, and newlines)
Scanner
的方法有以下几种:
方法名 | 解释 |
---|---|
hasNext() |
如果输入中还有小节,返回true |
next() |
返回输入中的下一个string 小节。若没有小节,返回错误 |
hasNextType() |
如果输入中还有对应的Type 类的小节,返回true 。Type 类可为Boolean Byte Double Float Int Long Short |
nextType() |
返回输入中的下一个Type 类小节。若没有小节或者小节不是Type 类,返回错误 |
hasNextLine() |
如果输入中还有另外一行文本,返回true |
nextLine() |
返回一整行的输入到回车结束(包括空格),并把光标移到下一行的开头 |
findInLine(Stirng s) |
尝试寻找输入的一行中是否有给定字符s 。 若有则返回此字符并把光标移到此字符后,若无则返回null 并且不移动光标。 |
参考
B. Eckel, Thinking in java. Upper Saddle River, N.Y. Prentice Hall, 2014.
M. Goodrich, R. Tamassia, and A. O’reilly, Data Structures and Algorithms in Java, 6th Edition. John Wiley & Sons, 2014.
Java转义字符, 知乎专栏. https://zhuanlan.zhihu.com/p/30158394 (accessed Sep. 14, 2021).
写在最后
Java相关的基础知识十分重要,会继续学习,继续更新.
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

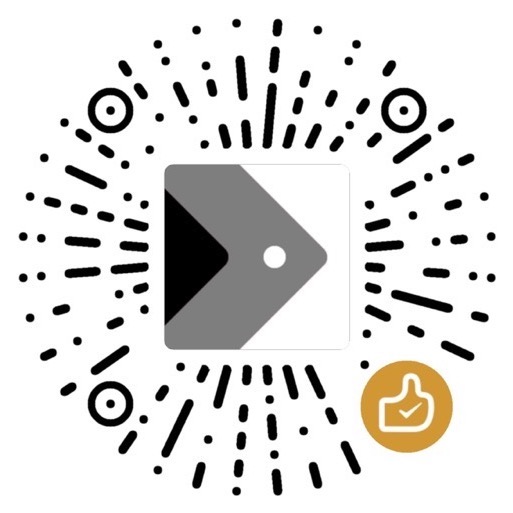