Java Iterator - Java 迭代器
Made by Mike_Zhang
Java主题:
1. Intro
Let’s first have a look at the example code of the following program:
1 |
|
Output:
1 |
|
It has a classical For-Each
loop in Java. But why Java knows how to iterate through the ArrayList based on for (int j : arr)
?
Actually, Java has compiled the above loop part code into:
1 |
|
With the help of the Iterator
class, Java can reach each element in the ArrayList, as well as know the next element of current one in the sequence.
An iterator is a soft design pattern that describe the process of scanning through a sequence of elements, once element a time, in which the element includes container, streaming from network, or a serial of computation, etc.
In Java, iterator is abstracted in java.util.Iterator
interface.
2. Iterator Interface
There are three methods in the java.util.Iterator
interface:
At the beginning, there is a reference points to the first element in the sequence.
hasNext()
: Returntrue
if the current reference has a next element, otherwise returnfalse
.next()
: Return the current pointing reference and update the reference to the next element.remove()
: Remove the element that returned by the most recently called bynext()
method. Return exception if that element has already been removed or no element has been returned bynext()
method.
With first two method, we can use general loop to iterate through the iterator sequence.
For a Iterator<Integer> i
1 |
|
The above code will print out the sequence of elements in the iterator i
.
3. Iterable Interface
Iterator
contains the content of the iterator sequence to be processed, as well as some method to control the iterator. One iterator instance only supports once traversal, and there is not way to restart the iterator from the beginning.
However, multiple iteration is commonly used in program.
Iterable
Interface can help us to create more new iterator instances for multiple iteration, which describes the ability of a class to be iterated.
The Iterable
interface contains only a single method:
iterator()
: Return aniterator
instance of collection.
Once the method iterator()
is called, a new Iterator instance will be created and returned.
One of the most important usage of Iterable
interface is the For-Each
loop in Java.
Any instance or collection of a class implementing the Iterable
interface can be iterated in the For-each loop.
1 |
|
where
ElementType
is the type of the element in the instance or collection, which is returned bynext()
method in theIterator
interface;variable
is one element with the type ofElementType
in the collection;collection
is the instance or collection of the class implementing theIterable
interface.
Above code can be translated into:
1 |
|
4. Iterator Implementation
With the help of the Iterable
interface, any class implemented the Iterable
interface can be iterated easily after creating instances implemented iterator
interface.
However, there two different way to implement an iterator
interface:
- snapshot iterator; and
- lazy iterator.
The mainly different if whether the subsequent changes in the collection will affect the iterator or not.
snapshot iterator:
- Initially, maintains a private copy of the primary collection of elements. All traversals are based on that copy, which will not be affected by any subsequent changes, due to the primary collection is immutable.
- Since a copy need to be made at the beginning, this approach is slow as running in the $O(n)$ time.
lazy iterator:
- No copy is made. It performs a piecewise traversal when
next()
is called based on the current content of the collection. So the lazy iterator is fast (in $O(1)$) but will be affected by any subsequent changes in the collection.
- No copy is made. It performs a piecewise traversal when
Following are the snapshot iterator Implementations with ArrayList
and LinkedPositionalList
.
4.1 Implementation with ArrayList
Refer to the original ArrayList in previous post.
Key steps:
- Make the
ArrayList
implement theIterable<E>
interface (default in Java); - Add a
iterator()
method to theArrayList
class to return anIterator
instance; - Add a
ArrayIterator
inner class to theArrayList
class to implement theIterator
interface.
Java Implementation:
1 |
|
Test:
1 |
|
Output:
1 |
|
4.2 Implementation with LinkedPositionalList
Refer to the original LinkedPositionalList in previous post.
Due to the LinkedPositionalList uses Position
ADT, its iterator can be implemented in two different ways: iterate the elements or iterate the positions.
We have both of them:
We will define the
iterator()
method return the iterator ofelement
in the LinkedPositionalList. In addition, a newpositions()
method will be added to return the iterable instance ofPosition
in the LinkedPositionalList.
Key steps:
- Make the
LinkedPositionalList
implement theIterable<E>
interface; - Add a
PositionIterator
inner class to theLinkedPositionalList
class to implement theIterator
interface, in order to realize all iterator functions on the position of the elements; - To let our
positions()
method return a Iterable instance on position, we need to add aPositionIterable
inner class implementing theIterable
interface, in which we add aiterator()
method to return aPositionIterator
instance. Afterwards, thepositions()
method will return aPositionIterable
instance. (What a perfect encapsulation!!! Why? The most outer classLinkedPositionalList
implements the Iterable interface but returning the element, so we have a new Iterable class to return position, so the PositionIterable comes!); - Add a
ElementIterator
inner class to theLinkedPositionalList
class to implement theIterator
interface to realize all iterator functions on the element of the sequence, which only need to adapt from thePositionIterator
class by returning the element of the position. - Add a
iterate()
method in theLinkedPositionalList
class to return anElementIterator
instance for the iterator of elements.
Java Implementation:
1 |
|
Test:
1 |
|
Output:
1 |
|
References
Goodrich, M., Tamassia, R., & O’reilly, A. (2014). Data Structures and Algorithms in Java, 6th Edition. John Wiley & Sons.
写在最后
Java相关的基础知识十分重要,会继续学习,继续更新.
最后,希望大家一起交流,分享,指出问题,谢谢!
原创文章,转载请标明出处
Made by Mike_Zhang

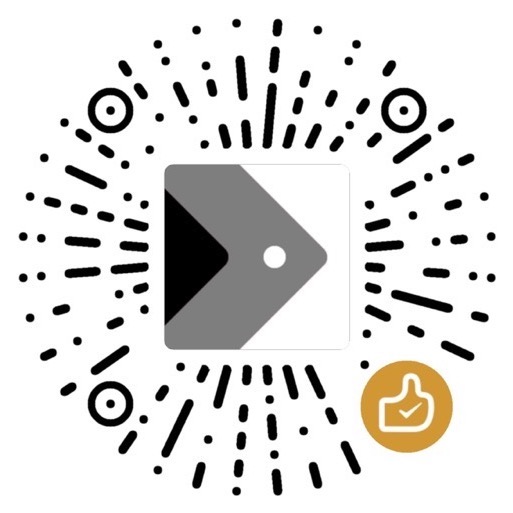